Java is a dynamic OOP language that allows data structures like Lists, arrays, variables, and many more to store data. Each created data structure has a specific data type allowing users to enter only specific types of data like numbers or characters. However, with the utilization of the parent data type “Object” we can store multiple types of data.
Among values, the “null” value is more popular because of its non-existence or holding of no value. Moreover, it cannot be assigned to primitive data types like “int”, “char”, “long”, and “double” except for “String”.
This guide covers the following sections:
- Method 1: Check If an Object is Null Using the “==” Operator
- Method 2: Check If an Object is Null Using the “Objects.isNull()” Method
- Method 3: Check If an Object is Null Using the “Optional” Class
- Method 4: Check If an Object is Null Using the “requireNonNull()” Method
- Method 5: Check If an Object is Null Using the Stream API
- Method 6: Check If an Object is Null Using the Apache Commons Lang3
How to Check if an Object is Null in Java?
The Object for nullability(holding null as a value) is checked to ensure that our targeted data structure has some value or not. This checking allows users to identify whether to perform desired operations over that data structure or not. This process is usually done while taking user inputs, to ensure the user has entered some data for required fields. After checking, operations like the verification or validation of the data are performed.
Let’s walk through various methods to identify if an Object is null or not in Java.
Method 1: Check If an Object is Null Using the “==” Operator
The “==” operator compares the references of both operands to check whether they are equal or not. It returns the result in boolean values and the user can display custom messages as well using the conditional statements. For instance, consider the below-mentioned code:
public class Nullability {
public static void main(String[] args) {
Object Obj = null;
if(Obj == null)
{
System.out.println("\nThe Provided Object 'Obj1' holds a null value");
}else {
System.out.println("\nThe Object 'Obj1' do not hold a null value");
}
}
}
In the above code block:
- First, create an Object with the value of “null”. This Object is then compared with the “null” value using the “==” operator along with the “if/else” statements.
- The “if/else” statements display a message over the console according to the result provided by the “==” operator.
Output:

Method 2: Check If an Object is Null Using the “Objects.isNull()” Method
The “Object” class provides an “isNull()” method to check the nullability of provided data structures. In our case, the “isNull()” method is used with the “for” loop to check the nullability for array elements:
import java.util.*;
public class Nullability {
public static void main(String[] args) {//Setting up the targeted List
Object[] demo = {"hello", 15, null, 7.77};
for (int i = 0; i < demo.length; i++) {
if(Objects.isNull(demo[i]))
{
System.out.println("\nThe Object Element at Index "+ i +" Contains a Null Value");
} else {
System.out.println( "\nThe Object element at index " + i + " do not contain a null value" );
}
}
}
}
The above code block works like this:
- First, declare an “Object” type array named “demo” containing multiple elements and utilize the “for” loop to iterate over this array.
- In each iteration, the nullability for a single array element is checked using the “Objects.isNull()” method. Also, display the corresponding message on the console.
The below output shows the nullability results for each array element:
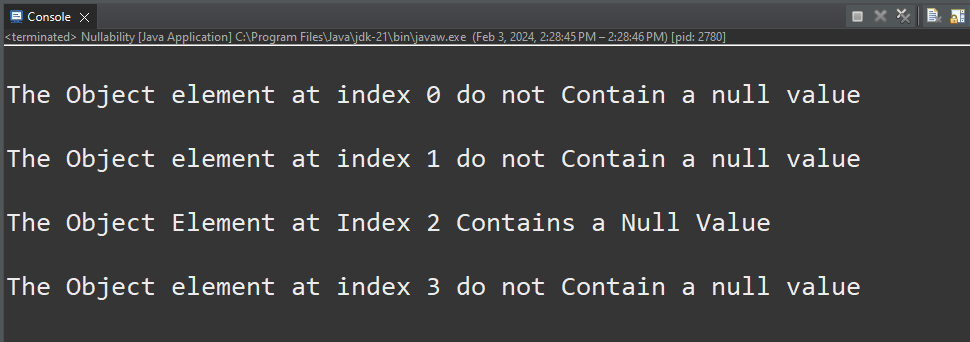
Method 3: Check If an Object is Null Using the “Optional” Class
The “Optional” class is available in the Java 8 or above versions. Its main purpose is to identify the nullability in the provided data Structure. In case the data structure element contains a “null” value, then it will be selected by the “isPresent()” method. The selected element is returned using the class “get()” method. It also offers the “orElse()” method to perform specific operations when a “null” value is not found.
Let’s proceed to the below code block for practical demonstration:
public class Nullability {
public static void main(String[] args) {
long endTime, startTime;
Object demoObj = null;
startTime = System.nanoTime();
Optional<Object> optionalStr = Optional.ofNullable(demoObj);
if (optionalStr.isPresent()) {
System.out.println("Provided Object is not null: " + optionalStr.get());
} else {
System.out.println("Provided Object is null");
}
endTime = System.nanoTime();
System.out.println("\nExecution Time Using Optional API -> " + (endTime - startTime));
}
}
The internal working of the above code is as follows:
- First, declare two “long” type variables “endTime” and “startTime”. Also, create an Object “demoObj” with the value of a “null”. Now, call the “System.nanoTime()” method and store its value in the “startTime” variable.
- Next, pass the “demoObj” in an “ofNullable()” method and store the result in an “Optional” class instance named “optionalStr”.
- Then, attach the “isPresent()” method with “optionalStr” to check the existence of “null” values. In the case of true, the value is retrieved and displayed via the “get()” method.
- After that, call the “System.nanoTime()” method to retrieve the current time.
- Finally, subtract this time from the one stored in the “startTime” variable to retrieve the execution time.
Output
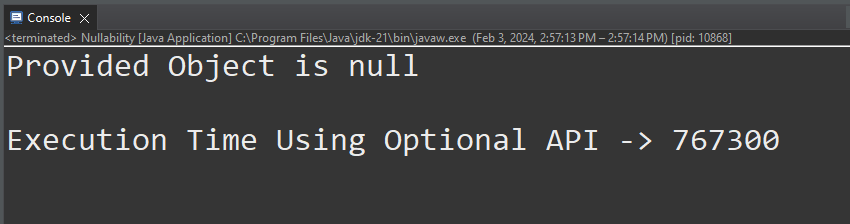
Method 4: Check If an Object is Null Using the “requireNonNull()” Method
The “requireNonNull()” method throws a “NullPointerException” whenever the “null” value is identified. Pass the variable or data structure to be checked for nullability as this method argument. If there is no element having a “null” value, then the exception will not be thrown and the remaining code gets executed:
import java.util.*;
public class Nullability {
public static void main(String[] args) {
//Defining the targeted Array named demo
Object[] demo = {"hello", 15, null, 7.77};
for (int i = 0; i < demo.length; i++) {
Objects.requireNonNull(demo[i], "Array Contains a NUll Element at index => " + i);
}
}
}
The explanation of the above code is as follows:
- First, create an Object type array named “demo” having multiple types of data.
- Then, utilize the “for” loop that traverses over each element of an array. Inside the loop, pass each element as the first argument of the “requireNonNull()” method and insert dummy data as a second argument.
- This method searches for a null value and throws an exception along with the data in the second argument.
Output
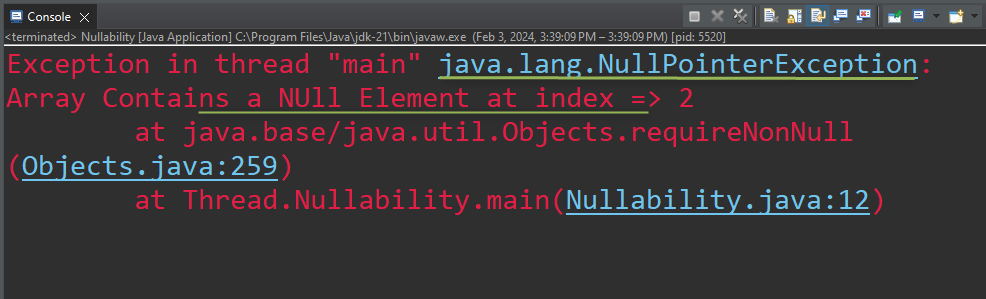
Method 5: Check If an Object is Null Using the Stream API
The “anyMatch()” and “allMatch()” methods of Stream API return “true” if any or all elements satisfy the condition respectively. Passing the condition of “null” as its argument, these methods can be used to identify whether some or all elements have the value of “null”. As shown below:
import java.util.*;
public class Nullability {//Initializing the “main()” method
public static void main(String[] args) {
//Setting up Object type targeted Array
List<Object> demo = Arrays.asList("hello", 15, null, 7.77);
boolean someNull = demo.stream().anyMatch(Objects::isNull);
boolean allNull = demo.stream().allMatch(Objects::isNull);
System.out.println("Some Elements are Null ->" + someNull);
System.out.println("All Elements are Null-> " + allNull);
}
}
The working of the above code is written below:
- First, create an Object type List “demo” containing multiple elements and send it over the stream using the “stream()” method.
- Then, apply the “anyMatch()” and “allMatch()” methods having the argument condition of Null over the “demo” List. Lastly, store and display the results of both methods.
Output
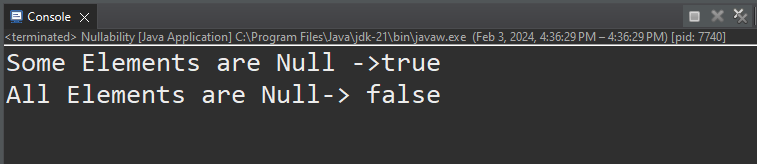
Method 6: Check If an Object is Null Using the Apache Commons Lang3
The “Apache Commons Lang3” is a third-party API that offers various classes and underlying methods to perform multiple operations. For instance, use its“ObjectUtils” class to perform operations related to Objects. This class offers an “allNull()” method that returns true if all elements of the provided collections are null or vice versa.
To use the “Apache Commons Lang3” API, insert the below-mentioned code inside the “pom.xml” file. This file is auto-created during the creation of the “Maven” project:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.14.0</version>
</dependency>
Once all is done, take a look at the Java code to check if an Object is Null or not:
import org.apache.commons.lang3.ObjectUtils;
import java.util.*;
public class Nullability {
public static void main(String[] args) {
Object[] demoObj = {"hello", 15, null, 7.77};
System.out.printf("\nIs all Element of '" + Arrays.toString(demoObj) + "' are Null => " + ObjectUtils.allNull(demoObj));
demoObj = new Object[]{null, null, null};
System.out.printf("\nIs all Element of '" + Arrays.toString(demoObj) + "' are Null => " + ObjectUtils.allNull(demoObj));
demoObj = new Object[]{"", null, " ", null};
System.out.printf("\nIs all Element of '" + Arrays.toString(demoObj) + "' are Null => " + ObjectUtils.allNull(demoObj));
demoObj = new Object[0];
System.out.printf("\nIs all Element of '" + Arrays.toString(demoObj) + "' are Null => " + ObjectUtils.allNull(demoObj));
}
}
The description of the above code is as follows:
- First, declare an array “demoIObj” having various types of data and pass it into the “allNull()” method. Also, pass it through the “Arrays.toString()” method to display the array elements over the console along with the result.
- Next, re-initialize the created array, and this time store only “null” elements in the array. Then, display the content of this array and pass them to the “allNull()” method to perform the required task.
- Repeat the same process with different values to grab all possible use cases.
Output
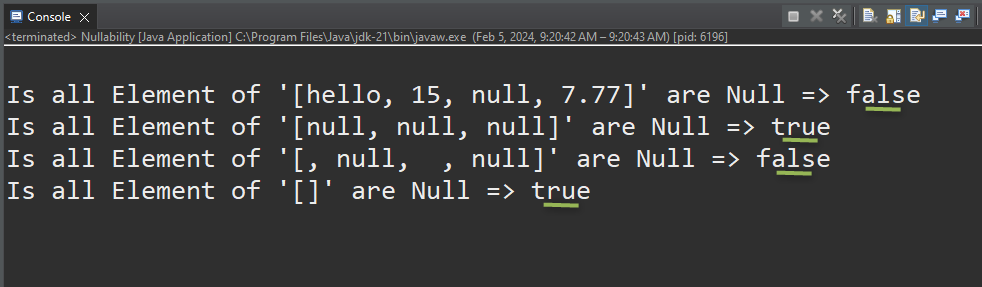
That’s all about checking the object for nullability in Java.
Conclusion
In Java, check if an Object is null or not using the “==” operator, “Objects.isNull()” method, “Optional” class, and “requireNonNull()” method. Especially for Java 8 or above versions, use the “Stream” API and “Apache Commons Lang3” library. All of these mentioned approaches provide easy identification for all or some Objects having the value of a null. However, as a recommendation according to ease of use, and optimized generation of a result the “Optional” class and “Apache Commons Lang3” Library should be used.