The CSV or “Comma Separated Values” is a file format containing data in rows and columns where each value is separated by a “comma” symbol. This file is mainly used to transfer data from one place to another due to its compressed size and easy-to-understand structure. The CSV file can be parsed, and modified using many programming languages like Java or Python. In Java, the common and optimized approach to parse a CSV file is using the third-party API named “openCSV”.
This guide explains the procedure to parse/read CSV files in Java by covering the following:
- How to Parse CSV Files in Java Using OpenCSV?
- How to Parse a CSV File Using Scanner Class?
- How to Parse a CSV File Using Split() Method?
How to Parse CSV Files in Java Using OpenCSV?
The “openCSV” is an open-source API that helps a lot during the CSV parsing phase. Moreover, it ignores commas in quoted elements, handles quoted entries, and allows the configuration of separator and quote characters. It works on a minimum of Java 8 version or Maven 3.
The openCSV provides several classes, which are utilized to work with CSV files and to perform multiple operations like importing, parsing, and so on. These classes are explained in below table:
Classes | Description |
---|---|
CSVReader | This class reads the CSV file as a String-type Array. It provides a lot of useful constructors to build a CSVReader like custom separator or quote characters. |
CSVReaderBuilder | This class extends the properties of an already created CSVReader object to add more functionality. Using it, the initial line number from where the parsing needs to start can also be set. |
CSVWriter | It allows users to insert custom separators or quote characters during the CSV file writing process. |
CsvToBean | Allows users to convert CSV files into Java beans or objects. |
BeanToCsv | This class allows users to export the created Java beans into CSV files. |
CSVParser | Accepts a single String and according to the delimiter token, quote, and escape characters perform the parsing. |
Prerequisites
To work with the “openCSV” API the user can either create a Maven or Java project according to their need:
- For Maven: create a new “Maven” project. Then insert the below-mentioned dependencies, inside its auto-created “pom.xml” file:
<dependency>
<groupId>com.opencsv</groupId>
<artifactId>opencsv</artifactId>
<version>4.1</version>
</dependency>
- For Pure Java: download the “jar” format file for “openCSV” from Apache Commons CSV. Then, place this file inside the Java project “lib” folder and set this file as a build path for your project. This build path is set by traversing the project properties and adding the downloaded jar file in the “Classpath” library option.
Once you’re done with the configuration, consider the below-stated examples to parse CSV files in Java via openCSV.
Example 1: Parse a CSV File Line by Line
The openCSV uses its “readNext()” method to parse the CSV File line by line. The enhanced for loop is also used to insert a specified token after each element. This token is inserted to enhance the readability of parsed data:
import java.io.*;
import com.opencsv.CSVReader;
public class Parser{
public static void main(String[] args){
try {//Parsing CSV File
FileReader filereaderObj = new FileReader("file.csv");
CSVReader csvReaderObj = new CSVReader(filereaderObj);
String[] record;
//Displaying parsed Data Line by Line
while ((record = csvReaderObj.readNext()) != null) {
for (String item : record) {
System.out.print(item + ", ");
}
System.out.println();
}
}//Handling Exceptions
catch (Exception error) {
error.printStackTrace();
}
}
}
In the above code:
- First, create an object “filereaderObj” for the “FileReader” class and pass the targeted CSV file in its constructor for parsing.
- Then, pass this “filereaderObj” object in the openCSV “CSVReader” class constructor to read the selected CSV file. Also, create an instance named csvReaderObj” for the “CSVReader“ class.
- Now, use the “while” loop that selects and stores each element of the “csvReaderObj” into the “record” List. The single parsed element is selected using the openCSV “readNext()” method.
- Finally, use the enhanced “for” loop to display the elements of a “record” List and add a random token(Comma “,”).
The generated output appears like this:
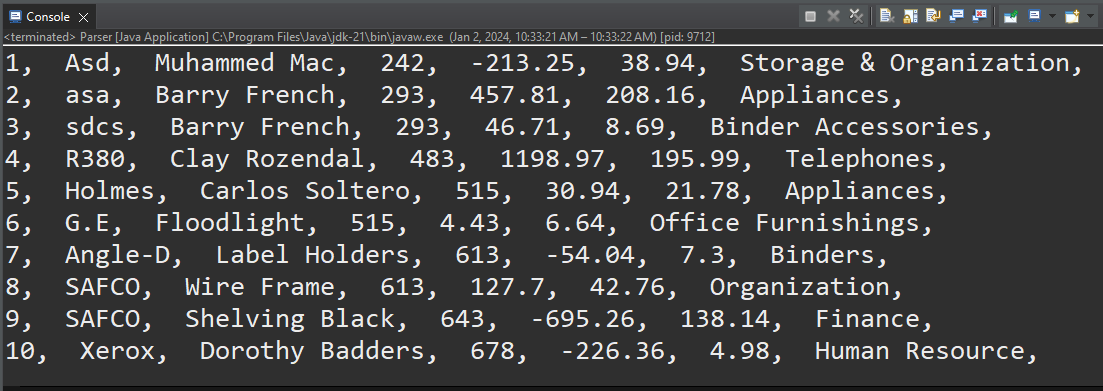
Example 2: Parse Specific Fields of a CSV File At Once
The “withSkipLines()” method skips/ignores the parsing of specific CSV fields. This method accepts an “integer” type value which tells how many CSV file rows need to be skipped from the top position:
import java.io.*;
import java.util.List;
import com.opencsv.CSVReader;
import com.opencsv.CSVReaderBuilder;
//Importing required Packages and classes.
public class Parser{
public static void main(String[] args){
try {
FileReader filereaderObj = new FileReader("file.csv");
CSVReader csvReaderObj = new CSVReaderBuilder(filereaderObj).withSkipLines(2)
.build();
List<String[]> record = csvReaderObj.readAll();
//Printing Parsed CSV File Data and Handling Exceptions
for (String[] csvRows: record) {
for (String item: csvRows) {
System.out.print(item + "\t");
}
System.out.println();
}
}
catch (Exception error) {
error.printStackTrace();
}
}
}
The above code works like this:
- First, select and read a targeted CSV file using the “FileReader” class.
- Next, apply the “withSkipLines()” method with the “CSVReaderBuilder” class to skip certain lines of the CSV file. Also, use the “build()” method to return the modified version of a read CSV file with the same return type of FileReader.
- Then, apply the “readAll()” method on the “csvReaderObj” to read all parsed CSV file data. Also, store this data in a String type List named “record”.
- Now, use the nested enhanced for loop to traverse through the List and display the parsed items on the console.
The generated output shows the parsing of a CSV file from the mentioned row:
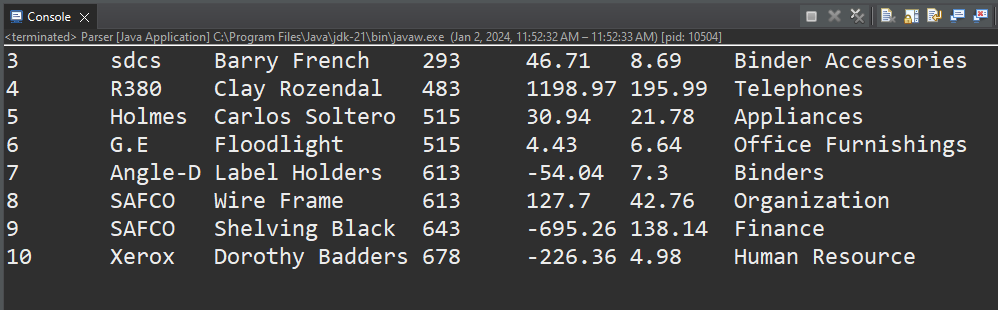
Example 3: Parsing Multiple CSV Files With Custom Separator
In this case, multiple CSV files get parsed using the functional approach, the custom separator is also inserted as a parser token:
package demo;
//Importing required Packages and openCSV classesimport java.io.*;
import java.util.List;
import com.opencsv.CSVParser;
import com.opencsv.CSVParserBuilder;
import com.opencsv.CSVReader;
import com.opencsv.CSVReaderBuilder;
public class Parser{
public static void Parsing(String csvfile) {
try {//Parsing the CSV File
FileReader fileReaderObj = new FileReader(csvfile);
CSVParser parser = new CSVParserBuilder().withSeparator(',').build();
CSVReader csvReaderObj = new CSVReaderBuilder(fileReaderObj).withCSVParser(parser).build();
List<String[]> record = csvReaderObj.readAll();
//Displaying parsed CSV File data
for (String[] csvRows : record) {
for (String item : csvRows) {
System.out.print(item + " <+> ");
}
System.out.println();
}
}
catch (Exception error) {
error.printStackTrace();
}
}
public static void main(String[] args){
//Passing Multiple Files to get Parse
System.out.print("Parsing First CSV File: \n");
Parsing("random.csv");
System.out.print("\n\nParsing Second CSV File: \n");
Parsing("file.csv");
}
}
In the above code snippet:
- First, define a “Parsing()” method inside the “Parser” class which accepts a single parameter of the targeted CSV file named “csvfile”.
- Now, pass the CSV file to the “FileReader” class constructor to read its content.
- Then, create an object for the “CSVParserBuilder” class to add a custom Separator for parsing.
- Also, apply the “withSeparator()” method with the parameter of “,” and “build()” method to it. This parameter of comma “,” allows you to modify the custom separator token symbol.
- Now, create an object “csvReaderObj” for the “CSVReaderBuilder” class and pass “fileReaderObj” to its constructor.
- After that, apply the “withCSVParser()” having the parameter of the “parser” object and “build()” methods for parsing.
- In the end, invoke the “Parsing()” method inside a “main()” method and pass random CSV files for parsing. These random CSV files in our case are “random.csv” and “file.csv”.
The generated output shows the parsed CSV file data:
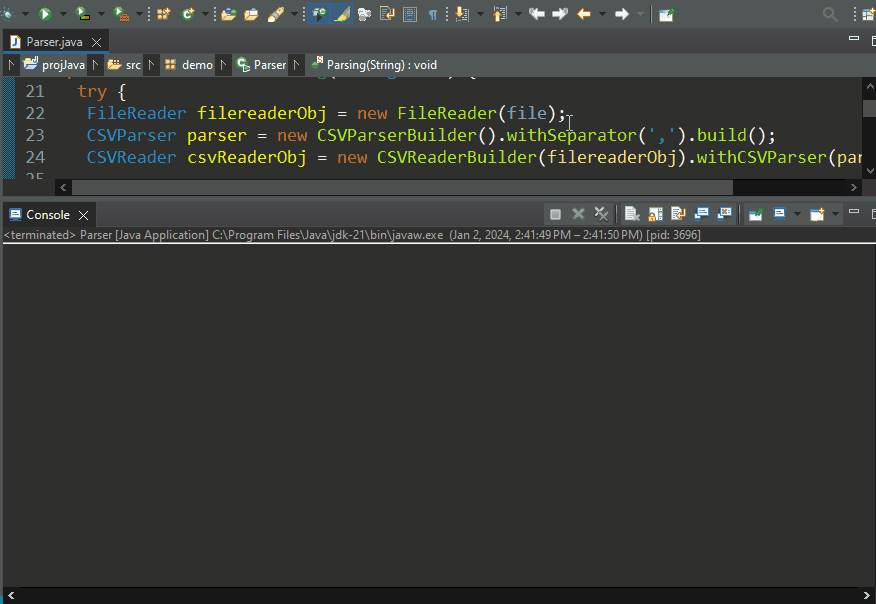
How to Parse a CSV File Using Split() Method?
The “split()” method uses the “delimiter” as a regex expression to parse the provided CSV file. The specific symbol(comma “,” ) is passed as a delimiter for CSV files because each value in the CSV file is separated by a comma. The “bufferReader” class is also utilized to read the CSV file line by line using the “readLine()” method:
import java.io.*;
public class Parser{
//defining the main() method.
public static void main(String[] args){
System.out.print("\n\nParsed CSV File: \n");
Parsing("file.csv");
}
public static void Parsing(String csvFile) {
String csvline = "";
String splitSymbol = ",";
try
{
BufferedReader bufferReadObj = new BufferedReader(new FileReader(csvFile));
while ((csvline = bufferReadObj.readLine()) != null)
{
String[] column = csvline.split(splitSymbol);
//Custom String to display parsed CSV data
System.out.println("Employee No " + column[0] + ": \n[ Invention = " + column[1] + ","+ " Creater Name = " + column[2] + ", \nBranch Number = " + column[3] + "," + " First year Income = " + column[4] + ", Last year Income = " + column[5] + "," + " Dept = " + column[6] +" ]\n");
}
bufferReadObj.close();
}
catch (IOException excep)
{
excep.printStackTrace();
}
}
}
The above code works like this:
- First, pass the CSV file path as an argument in the custom-defined “parsing()” method to parse the provided CSV file.
- Then, define a String type empty variable “csvLine” and “splitSymbol” variable with the value of a comma “,”.
- Next, create an object for the “BufferReader” class and pass the CSV file as its constructor to read the file content.
- Then, split the CSV file in the rows and columns format whenever the “splitSymbol” appears to parse.
- Store this split or parsed CSV data in another List named “column”.
- Finally, select and display each column of the original parsed CSV file on the console using their indexes.
The output shows the parsed form of a provided CSV file in Java:
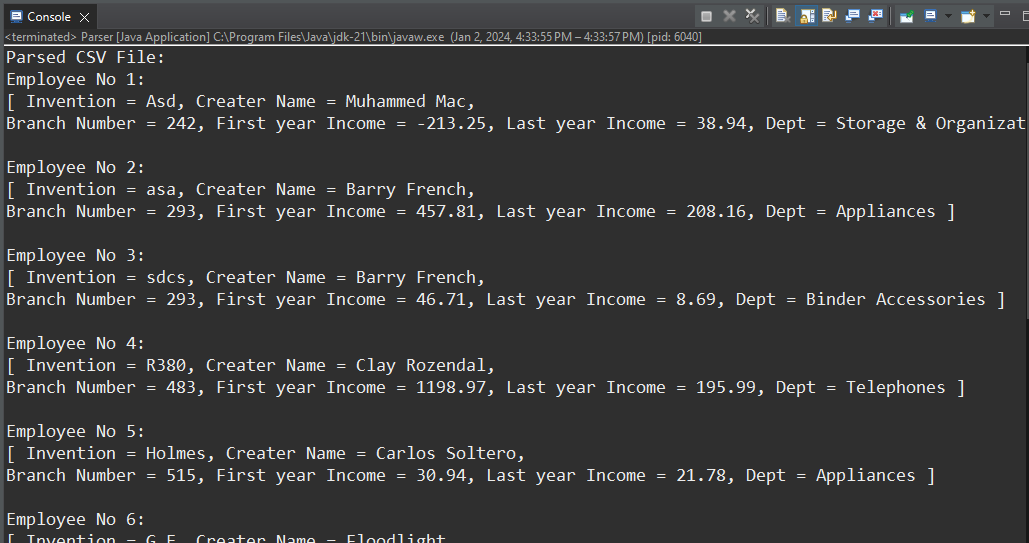
How to Parse a CSV File Using Scanner Class?
In Java, the Scanner classes use the combination of “useDelimiter()”, “hasNext()” and “next()” methods to parse a single provided CSV file:
import java.io.*;
import java.util.*;
public class Parser{
public static void main(String[] args){
System.out.print("Parsed CSV File: \n");
Parsing("file.csv");
//passing CSV File to get parse
}
public static void Parsing(String file) {
try
{
Scanner scanObj = new Scanner(new File("file.csv"));
scanObj.useDelimiter(",");
while (scanObj.hasNext())
{
System.out.print(scanObj.next() + " => ");
}
scanObj.close();
}
//handling exceptions
catch (IOException excep)
{
excep.printStackTrace();
}
}
}
The description of the above code is as follows:
- Initially, create an instance “scanObj” for the “Scanner” class and pass the CSV file in its constructor.
- Then, use the “scanObj” to invoke its “useDelimiter()” method having the parameter of a “Comma (,)” symbol.
- After that, utilize a “for” loop that iterates till “scanObj” contains a value. Also, use the “hasNext()” method to check the existence of upcoming values.
- Inside the loop, select the “next” upcoming values and print them over the console along with a custom token symbol.
The output confirms the parsing of a provided CSV file in Java:
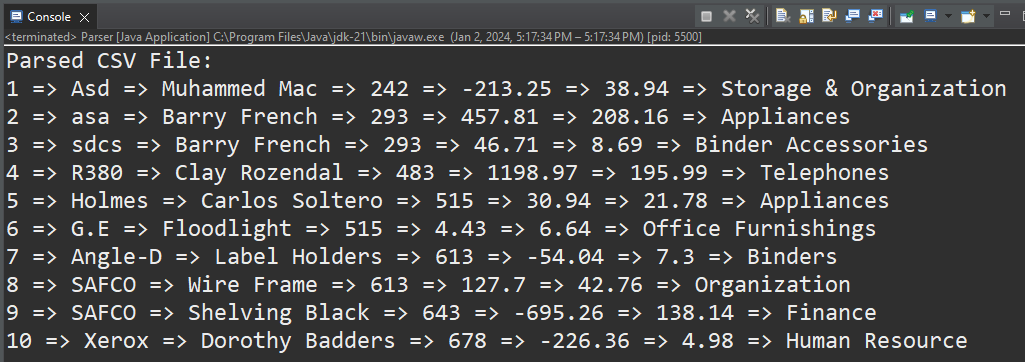
That’s all about the parsing of CSV files in Java using openCSV.
Conclusion
The “openCSV” library provides full control over the parsing procedure to parse a CSV file in Java. Due to its ease of usability and the availability of predefined methods. For parsing the CSV files, “CSVParser, “CSVParserBuilder”, “CSVReader”, and “CSVReaderBuilder” classes are mostly used. Alternatively, the user can use the “Scanner” class and “Split()” Method to parse CSV files in Java. With the utilization of discussed OpenCSV classes and alternative approaches, the user can easily parse CSV files in Java.