Strings are a sequence of characters commonly used in Python to work with textual data. In Python, strings can represent dates and times, however, Python provides a more appropriate data type called DateTime for working with dates and times. Moreover, strings can be converted into date time to perform various operations such as sorting, etc.
In this article, we will discuss the two commonly used methods for converting a string into a datetime object.
How to Convert a String into DateTime in Python?
There are several methods to convert a string into datetime. Some of them are:
- Using strptime() Method
- Using Parse() Method
- Using Third-Party Libraries
Let’s discuss them one by one.
Method 1: Use strptime() Method to Convert a String into DateTime in Python
Python supports a built-in method named “strptime()” for converting strings into datetime. We have to import the datetime class from the datetime module in order to do so. strptime() method converts date and time strings into objects. It takes 2 parameters. The first one is the string and the second one is the date time format specifier.
Note: The strings must be in a proper format in order to be converted to date-time objects, such as ( %b %d %Y %I:%M%p) where
- %b means the month of the year.
- %d represents the day of the month.
- %Y represents the year(must be in 4 digits).
- %I represent Hour(12 hour clock) as a decimal number.
- %M represents minutes as a decimal number.
- %p represents AM or PM.
Consider an example shown below:
from datetime import datetime
current_date = "Sep 07 2023 11:58AM"
datetime_object = datetime.strptime(current_date, '%b %d %Y %I:%M%p')
print(type(datetime_object))
print(datetime_object)
In the above code:
- The datetime class is imported from the datetime module.
- The next line defines a variable current_date which specifies the current date and time in a string format.
- strptime() method of the datetime class is used to parse the current_date string and convert it into a date-time object.
- The code then prints the type of the datetime object.
- Finally, The next line prints the date time object.
The output of the above code is as follows:
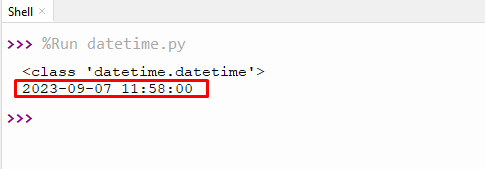
It can be seen that the current date and time are displayed.
Method 2: Use the Parse() Method to Convert a String into DateTime in Python
A string can also be converted into a date-time object using the parse() method. It takes only one argument which is the string. This method automatically detects and parses the date and time formats. Let’s see an example:
from dateutil import parser
date_time = parser.parse("Sep 07 2023 12:10PM")
print(date_time)
print(type(date_time))
In the above code:
- The parser class is imported from the dateutil module.
- In the second line, the parse() method of the parser class is used. This method parses the date and time formats.
- The next line prints the date time object.
- The code then prints the type of the datetime object.
The output of the above code is as follows:
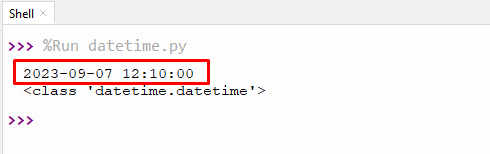
We can see that the current date and time are printed.
Method 3: Use Third-Party Libraries to Convert a String into DateTime in Python
Third-party libraries are also used to convert strings into datetime objects. Maya is a library that is used to parse strings and change time zones. Let’s see an example:
import maya
dt = maya.parse('2023-09-07T14:21:25Z').datetime()
print(dt.date())
print(dt.time())
print(dt.tzinfo)
In the above code:
- Maya library is imported.
- maya.parse function is used to parse the given string. It converts the given string into maya.MayaDT object and then the datetime function is called to convert it into a standard Python date time object.
- The date, time, and timezone are printed.
The output of the above code is as follows:
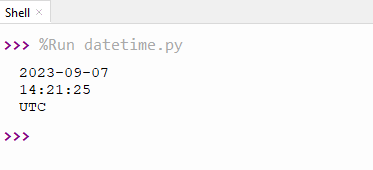
The date, time, and time zone are displayed successfully.
Conclusion
We can convert a string into datetime in Python using several methods. One such method is strptime() which parses the given string and converts it into a date time object. The second method is the dateutil module in which the parse() method of the parser class is used. This method can automatically parse the date and time formats.