Date is the most dependent element when it comes to the creation of applications like to-do lists, assistants, or calendars. The retrieval and customization of the current or provided date is very straightforward. However, it becomes a little messy when the developer wants to retrieve the current or provided date after the addition of specific days. Luckily! Java offers multiple approaches to add single or multiple days into the date which are going to be described in this article.
Content Overview
- How to Add Days to Date in Java?
- Method 1: Use of Calendar add() Method to Add Days to Date
- Method 2: Add the Day in a Date Using the Instant “plus()” method
- Method 3: Add the Day in a Date Using the LocalDate “plusDays()” method
- Method 4: Add the Day in a Date Using Apache Commons Library
- Bonus Tip: How To Add Working Days To A Date In Java?
How to Add Days to Date in Java?
Java offers different methods to add days to date for the generation of the required result. Moreover, they can add a single or multiple days to the current or provided date as per requirements. To skip certain days like weekends and add days only to the working days, the “DayOfWeek” class is very popular to use. These methods or approaches are explained below in detail.
Method 1: Use of Calendar add() Method to Add Days to Date
The “add()” method of a “Calendar” class allows the users to insert or remove days, months, or years from the provided Date. The addition of elements into the day/s does not break the structure of the Date. Moreover, the “add()” method adds a day to the date according to the calendar’s rules(day <=31, 30, or 28, month <=12, and, year should be a 4-digit value).
Let’s have a look at a couple of examples to add a day in the provided and current date using the “add()” method:
Note:
In the below couple of examples, the methods offered by the “ParseException” and “SimpleDateFormat” packages are used. These methods handle the exceptions related to the date parsing and specify the format for a generated date.
Example 1: Add a Day in the Provided Date Using Calendar “add()” Method
In this example, the provided date is incremented by a single and multiple days separately. For addition, the provided date is first parsed and then, the addition is performed on the day part. Let’s proceed toward the implementation of the discussed scenario:
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
//Importing Required Packages
public class Dateadder {
//Defining Custom Function to add days to date
public static String dateAddDays(String provDate, int dayToAdd) {
SimpleDateFormat dateFormat = new SimpleDateFormat("dd-MM-yyy");
Calendar calendarIns = Calendar.getInstance();
try{
calendarIns.setTime(dateFormat.parse(provDate));
}catch(ParseException e){
e.printStackTrace();
}
calendarIns.add(Calendar.DAY_OF_MONTH, dayToAdd);
String dateAfter = dateFormat.format(calendarIns.getTime());
return dateAfter;
}
public static void main(String[] args) {
String provDate = "23-12-2023";
System.out.println(provDate + " <- Provided Date\n");
String modifiedDate1 = dateAddDays(provDate, 1);
System.out.println( modifiedDate1 + " <- Date After Adding 1 Days.");
String modifiedDate2 = dateAddDays(provDate, 5);
System.out.println( modifiedDate2 + " <- Date After Adding 5 Days.");
}
}
The description of the above code snippet is written below:
- First, define a function “dateAddDays()” that accepts two parameters of date “provDate” and day “dayToAdd”.
- Inside it, define the format for a date using the “SimpleDateFormat()” method and store it in a “dateFormat” object. Also, create an instance of the “Calendar” class by calling the “getInstance()” method.
- Then, utilize the “try/catch” block to perform the parsing of a provided date “provDate” and to handle the exception that may raised during the date parsing.
- Now, apply the “add()” method over the parsed date “calendarIns” and pass the “Calendar.DAY_OF_MONTH” to modify the day. Also, pass the “dateToAdd” as a second augment, it is the number of days to be added to the provided date.
- Define a “main()” method and a custom date such as “23-12-2023”. After that, pass the defined date and the number of days “1” and “5” to be inserted into the “dateAddDays()” method.
- Finally, store the result in a new String and display it on the console.
The generated output confirms the addition of a provided number of days in the date:
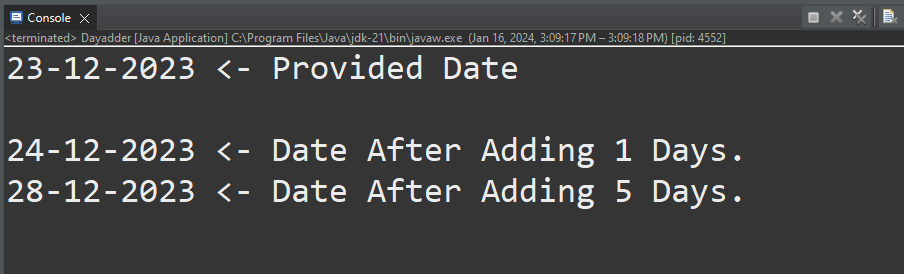
Example 2: Add a Day in the Current Date Using Calendar “add()” Method
In the below code block, single and multiple days are inserted into the current date:
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
//Importing Required Packages
public class Dateadder{
//Defining Custom Function to add days to date
public static String dateAddDays(int dayToAdd) {
SimpleDateFormat dateFormat = new SimpleDateFormat("dd-MM-yyy");
Calendar calendarIns = Calendar.getInstance();
System.out.println(dateFormat.format(calendarIns.getTime())+" <- Current Date");
calendarIns.add(Calendar.DAY_OF_MONTH, dayToAdd);
String modifiedDate = dateFormat.format(calendarIns.getTime());
return modifiedDate;
}
public static void main(String[] args) {
String modifiedDate1 = dateAddDays(1);
System.out.println( modifiedDate1 + " <- Date After Adding 1 Days.");
String modifiedDate2 = dateAddDays(5);
System.out.println( modifiedDate2 + " <- Date After Adding 5 Days.");
}
}
The working of the above code is as follows:
- First, define a method “dateAddDays()” that accepts the single parameters of days “dayToAdd”. In it, declare a date format using the “SimpleDateFormat()” method and create a “Calendar” class instance. Also, display the current date on the console.
- Next, invoke the “add()” method and pass the “Calendar.DAY_OF_MONTH” and “dayToAdd” parameters. This retrieves our required date with the addition of the provided days.
- Now, place the retrieved date in a new String “modifiedDate” and return it using the “return” keyword.
- In the end, call the “dateAddDays()” method and pass different integers as days to be inserted into the date. Display the result of these methods on the console as a final result.
Output:
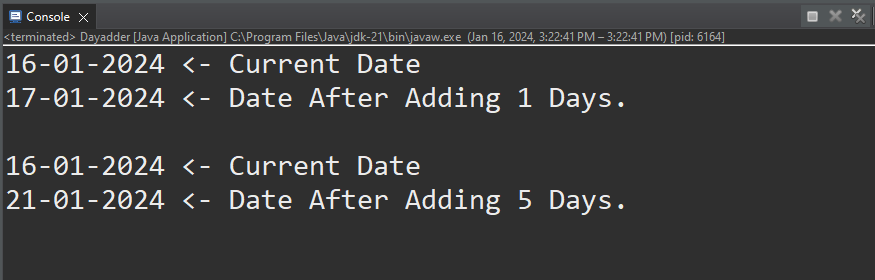
Method 2: Add the Day in a Date Using Instant “plus()” Method
The “plus()” method of an “Instant” class returns the duplicated version of the instant with the number of units or values added. The units in our case are the number of days to be inserted in the date. Along with it, the “plus()” method accepts a TemporalUnit as a second parameter that is set to the “ChronoUnit”. The “ChronoUnit” represents a set of date period units that help in the manipulation of date and time:
import java.time.Instant;
import java.time.temporal.ChronoUnit;
import java.util.Date;
//Importing Required Packages
public class Dateadder {
public static void main(String[] args) {
Date date = new Date();
Instant currDate = date.toInstant();
System.out.println("Current Date -> " + currDate);
Instant firstDate = currDate.plus(1, ChronoUnit.DAYS);
System.out.println("\nAfter Adding '1' Day: " + firstDate);
Instant secondDate = currDate.plus(5, ChronoUnit.DAYS);
System.out.println("After Adding '5' Days: " + secondDate);
}
}
The explanation of the above code:
- First, create an object of the “Date” class to extract the current date and time. Also, apply the “toInstant()” method on this object to select and store the date and time at that instant.
- Next, invoke the “plus()” method and pass the parameters of “1” and “ChronoUnit.DAYS” to add one day to the current date.
- In the same manner, call the “plus()” method and pass “5” and “ChronoUnit.DAYS” to add five days to the current date.
- In the end, store the resultant dates in separate “Instant” type variables namely “firstDate” and “secondDate”. Then, display them on the console.
Output:
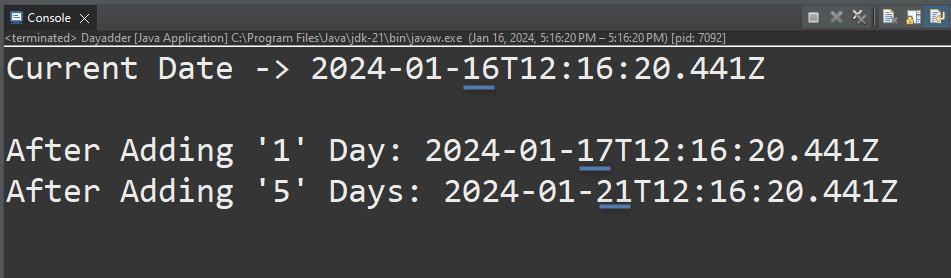
Method 3: Add the Day in a Date Using the LocalDate “plusDays()” method
In Java, the “LocaleDate” is an immutable class that represents the date in a default format of “yyyy-mm-dd”. It offers several methods to retrieve and modify the part or whole date effortlessly. The “plusDays()” method is one of these methods. The “plusDays()” efficiently adds days to the current or provided date and returns the modified version of the original date.
Take a look at the below code for practical implementation:
import java.time.LocalDate;
import java.util.Scanner;
public class Dayadder {
public static void dateAddDays(int year, int month, int day, int addDays) {
//Adding days in Provided Data
LocalDate fromProvDate = LocalDate.of(year, month, day).plusDays(addDays);
System.out.println(fromProvDate + " <- After Adding Days in Provided Date\n");
//Retrieving the Current Date
LocalDate currDate = LocalDate.now();
System.out.println(currDate + " <- Current Date");
//Adding days from Current Data
LocalDate fromCurrDate = currDate.plusDays(addDays);
System.out.println(fromCurrDate + " <- After Adding Same Days in Current Date");
}
public static void main(String[] args) {
Scanner myObj = new Scanner(System.in);
System.out.println("Enter Year");
int year = myObj.nextInt();
System.out.println("Enter Month");
int month = myObj.nextInt();
System.out.println("Enter Day");
int day = myObj.nextInt();
System.out.println("Enter Number of Days to Add");
int addDays = myObj.nextInt();
System.out.println(year + "-" + month + "-" + day + " <- User-Provided Date");
dateAddDays(year, month, day, addDays);
}
}
The working of the above code is written below:
- First, create a method “dateAddDays()” that accepts four integer type parameters namely “year”, “month”, “day”, and “addDays”. These parameters store the user-provided date and the days that need to be added to this date.
- Next, pass the “year”, “month”, and “day” in a “LocalDate.of()” method to create an instance of LocalDate from the passed date.
- Also, apply the “plusDays()” method and pass the parametric “addDays” variable to add this variable value(days) to the created date.
- Then, invoke the “localDate.now()” method to retrieve the current date and store its instance in a localDate type variable “currDate”.
- Now apply the “plusDays()” method on “currDate” and pass the “addDays” to add days into the “currDate” and display the result.
- Define the “main()” method and create an instance of the “Scanner” class. Next, retrieve the integer-based values from the user at runtime from the console using the Scanner “nextInt()” method. The retrieved values will be for the year, month, day, and days to be added.
- In the end, pass these values into the “dateAddDays()” method to add days to the provided and current date.
The below gif confirms the retrieval of a Date at runtime and the addition of days in a provided and current date:
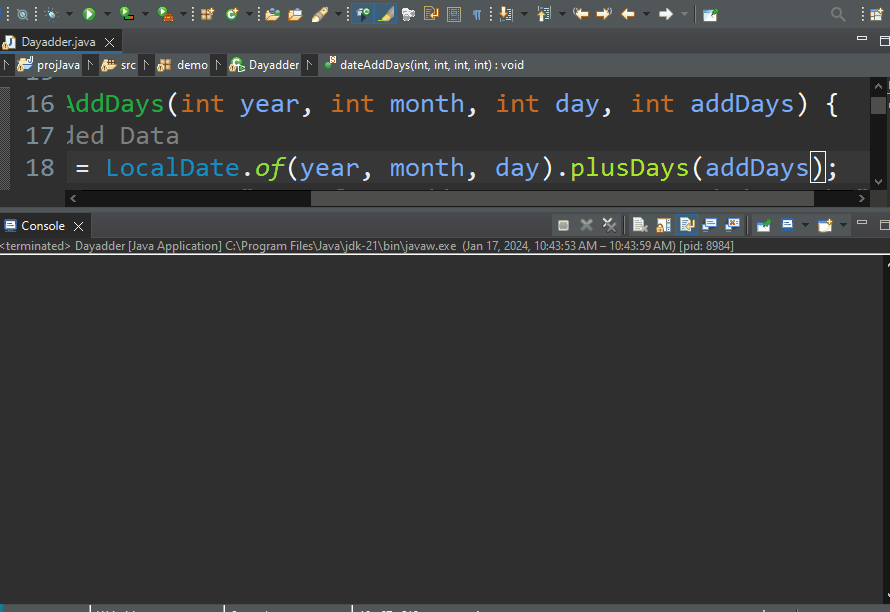
Bonus Tip:
Use the “plusMonths()”, “plusYears()”, and “minusDays” methods of LocalDate class to add months, years, or subtract days from the date.
Method 4: Add the Day in a Date Using Apache Commons Library
The Apache Commons library creates and maintains the reusable components. It contains several methods to deal with specific operations like adding days to date. To work with this library, the user can use the Maven project or Java project:
- For the Maven project, insert the below code in the automatically generated “pom.xml” file:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
- For Java, download the “Apache Commons” jar file and add that file as the “Classpath” for the “Java Build Path” of your project.
Once configured, place the below-mentioned code to add days to a current date:
import java.text.ParseException;
import java.util.Date;
import org.apache.commons.lang3.time.DateUtils;
//Importing the required Java Packages
public class Dayadder {
public static void main(String[] args) throws ParseException {
Date currDate = new Date();
System.out.println("Current Date: " + currDate);
Date upcomingDate = DateUtils.addDays(currDate, 6);
System.out.println("Date After Adding Days: " + upcomingDate);
}
}
The above code works like this:
- First, import the Apache Commons “DateUtils” and other packages via the import keyword.
- Then, create an object for the “Date” class and store it in a “currDate” variable.
- After that, pass this “currDate” and the number of days to be added in the Date as a parameter in the “DateUtils.addDays()” method.
- In the end, display the modified date on the console.
Output:
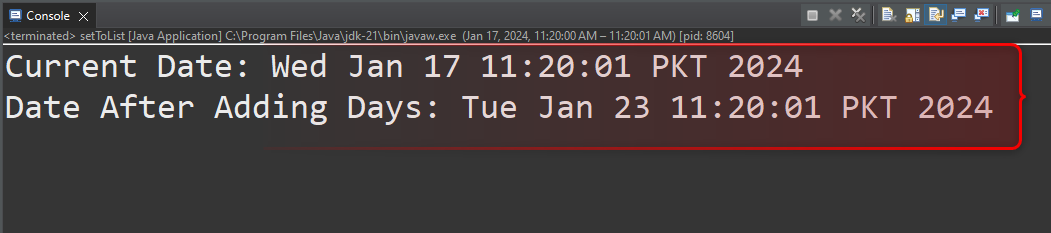
Bonus Tip: How To Add Working Days To A Date In Java?
In Java, the “DayOfWeek” class of the “time” package allows the user to add each day of the week separately. The user can apply custom logic on weekdays to set the working and weekend days. By combining it with the LocalDate “plusDays()” method, the days can be inserted only on the working days, as required. The implementation in Java is demonstrated below:
import java.time.LocalDate;
import java.time.DayOfWeek;
public class Dayadder {
public static void main(String[] args) {
LocalDate currDate = LocalDate.now();
int workDaysAdd = 7;
LocalDate resultDate = addWorkDays(currDate, workDaysAdd);
System.out.println("Date With Addition of Working Days: " + resultDate);
}
public static LocalDate addWorkDays(LocalDate currDate, int workingDays) {
int allDays = 0;
while (workingDays > 0) {
currDate = currDate.plusDays(1);
if (workDayChecker(currDate)) {
workingDays--;
}
allDays++;
}
System.out.println("Number of all Days: " + allDays);
return currDate;
}
public static boolean workDayChecker(LocalDate date) {
DayOfWeek singleDay = date.getDayOfWeek();
return singleDay != DayOfWeek.SATURDAY && singleDay != DayOfWeek.SUNDAY;
}
}
The above code works like this:
- First, create an “addWorkDays()” method accepting two parameters of date and working days to add. Inside it, add a single day into the provided date “currDate” using the “plusDays()” method and pass the date to a “workDayChecker()” method.
- Next, decrease the days to add “workingDays” by a factor of “1”, if the “workDayChecker()” method returns “true”. Also, increase the custom declared empty variable “allDays” to a factor of “1” and return the modified “currDate”.
- After that, define a boolean type “workDayChecker()” method which accepts a single parameter of “date”.
- Next, create a new instance of the “DayOfWeek” class for the provided “date” parameter. Then, use the “DayOfWeek” enumerations to check whether the current date is one of the working days or not and return the result accordingly.
- Inside the main() method, create a new object of LocalDate by applying the “now()” method to store the current date. Then, define a variable “workDaysAdd” and store the days “7” that need to be added to the current date.
- Now, pass the “currDate” and “workDaysAdd” variables into the “addWorkDays()” method to add days in the working days.
- In the end, display the resultant result on the console as the modified date.
The output shows that after adding the “7” days in the working days the “2024-01-26” date is generated. Along with it, total days along the weekend days, the “9” days are added:
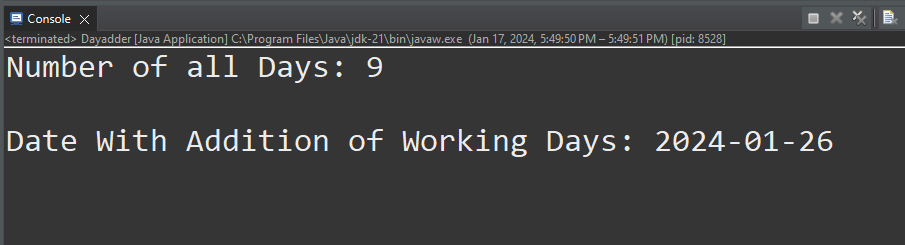
That’s all about adding days to date in Java.
Conclusion
In Java, the user can add days to date using the “add()” method of the Calendar class or the “plus()” method offered by the “Instant” class. To work with Java 8 or above versions, use the “plusDays()” method of the LocaleDate class or the “DateUtils.addDays()” Apache Commons Library. All of these methods provide full control over adding single or multiple days, months, or years in the provided or current date. Among these methods, the “plusDays()” is the recommended method due to its easy-to-understand working and availability for subtracting the days from Date. This guide has illustrated the procedure to add days to date in Java.