The dictionary is one of the Python data structures with a mutable property. The dictionary consists of “keys” and “values” enclosed by curly braces “{ }”. “Mutable” means we can change or modify the dictionaries by sorting the dictionary by a key or value, converting the dictionaries to JSON or any other format, extracting the targeted values or key information, or appending or removing the key values. However, dictionaries have ordered structures and do not allow duplicates.
This article is about sorting a dictionary by value in Python. However, the following topics will be elaborated in this context:
- How to Sort a Dictionary by Value in Python?
- Sort keys in the Dictionary
- Using “Sorted()” to Sort a Dictionary by Value
- Using “Sorted()” with “key” Parameter to Sort a Dictionary by Value
- Using “lambda” Function Aggregation With Sorted()
- Using Dict Comprehension Aggregation With “Argsort” Function
- Using Dict comprehension One-Liner approach With Lambda
- Using “Sorted()” With “itemgetter” Function
- Using “Sorted()” With the “zip” function to Sort a Dictionary by Value
- Defining a User-Defined Function to Sort a Dictionary by Value
- Bonus Tip: Change the Sorting Order of the Dictionary
How to Sort a Dictionary by Value in Python?
To sort data in a dictionary by values or keys utilize the sorted() standard built-in function in Python. However, there are many other prevalent methods to sort a dictionary by value in Python. Sorting a dictionary by value is beneficial in extracting and viewing numeric data like sorting the population of a particular state or viewing the population within the range. However, dictionaries can be converted to the DataFrame for further data handling and analysis.
Let’s understand the concept of sorting a dictionary by key in Python:
Sort keys in the Dictionary
To sort a dictionary by key in Python alphabetically, use the sort() function. The sort() function will sort the dictionary in alphabetical order by taking the “keys” as a reference. However, utilize the one-liner dict comprehension structure to search for the items in a dictionary and to iterate the sorting process over each key-value pair:
import pandas as pd
# considering dictionary
dict = {'city': ['?stanbul' ], 'population': ['16 million'], 'famous for': ['The Blue Mosque']}
print("\n Original Dict :\n", dict)
#converting dict to list
dict_sort_Keys = list(dict.keys())
#sorting the dictionary keys alphabetically
dict_sort_Keys.sort()
#using a one-liner dict comprehension approach
sorted_dict = {i: dict[i] for i in dict_sort_Keys}
#printing the sorted Dictionary
print("\nSort Dictionary by Keys alphabetically:\n", sorted_dict)
Output

Alphabetical Sorting via “OrderedDict” Module
Consider importing the module “OrderedDict”, it will sort the dictionary by key. To access the key-value pair in the dictionary, use the “dict.items()”. However sort the dictionary by targeting the key, utilizing the sorted() function. The “OrderedDict” package will remember the key that was first sorted.
Here’s how you can sort the dictionary by key in Python by utilizing the “OrderedDict” package:
import pandas as pd
# considering dictionary
dict = {'city': ['?stanbul' ], 'population': ['16 million'], 'famous for': ['The Blue Mosque']}
print("\n Original Dict :\n", dict)
# Creates a sorted dictionary (sorted by key)
from collections import OrderedDict
dictionarysort = OrderedDict(sorted(dict.items()))
print(dictionarysort)
#alphabetically sorting
print("\nSort Dictionary by Keys:\n", dictionarysort)
Output
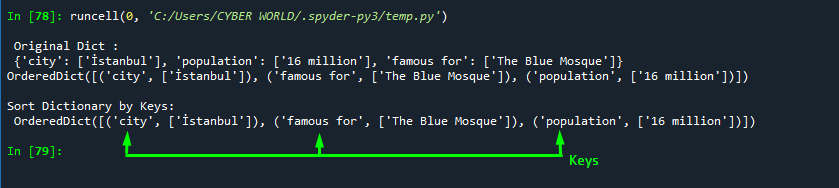
Now moving back to the topic and exploring the prevalent approaches to sort the dictionary by value in Python.
Approach 1: Using “Sorted()” to Sort a Dictionary by Value
By utilizing the sorted() function with the “values()” function, you can sort a dictionary by value in Python. To do so, invoke the “values()” function with a dictionary variable using the dot(.) function. However, the program will return the lists of the values enclosed by square brackets ([ ]).
This method is applicable where you want to grab the “values” from the dictionary:
import pandas as pd
import numpy as np
import operator
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
#dict values sorting
dictionarysort = sorted(dict.values())
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
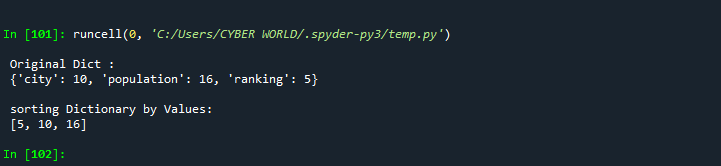
Approach 2: Using “Sorted()” With “key” Parameter to Sort a Dictionary by Value
Using a dictionary with the “get” attribute by the dot (.) operator, you can sort a dictionary by value in Python. To implement this, you need to specify the “dict.get” attribute within the “key” parameter of the sorted() function.
However, this method uses the for..in structure to access the key-value pair in the dictionary. The for…in non-list comprehension structure will unpack the key and their values from the sorted dictionary and return the key with the sorted value on the kernel console:
import pandas as pd
import numpy as np
import operator
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
print("\nsorting dict values:")
#dict values sorting
for i in sorted(dict, key=dict.get, reverse=False):
print( i, dict[i])
Output
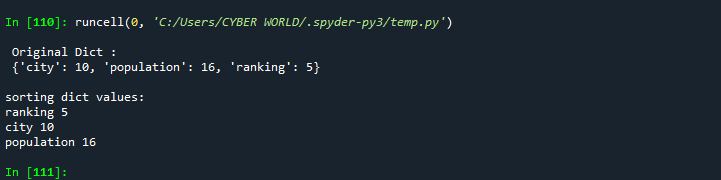
Approach 3: Using “lambda” Function Aggregation With Sorted()
The “OrderedDict” function will be utilized to sort a dictionary by value in Python. For sorting the dictionary by value, use the “lambda” operation on the key to iterate over the dict items:
import pandas as pd
# considering dictionary
dict = {'city': ['?stanbul' ], 'population': ['16 million'], 'famous for': ['The Blue Mosque']}
print("\n Original Dict :\n", dict)
from collections import OrderedDict
# dictionary sorted by value
dictionarysort = OrderedDict(sorted(dict.items(), key=lambda i: i[1]))
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
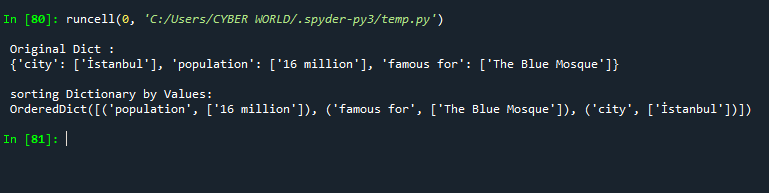
Approach 4: Using Dict Comprehension Aggregation With “Argsort” Function
Another prevalent approach to sorting a dictionary by value in Python uses the built-in functions of dictionary “values()” to access values in the key-value pair and “keys()” to access keys in the key-value pair of the dictionary. The “argsort” function will access read and sort the dictionary numeric values index-wise. However, utilize the dict-comprehension to iterate over the items in the key-value pair. This will return the output in dictionary format:
import pandas as pd
import numpy as np
from collections import OrderedDict
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
dict_keys = list(dict.keys())
dict_values = list(dict.values())
dict_value_index_sorting = np.argsort(dict_values)
#sort dict by value
dictionarysort = {dict_keys[i]: dict_values[i] for i in dict_value_index_sorting}
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
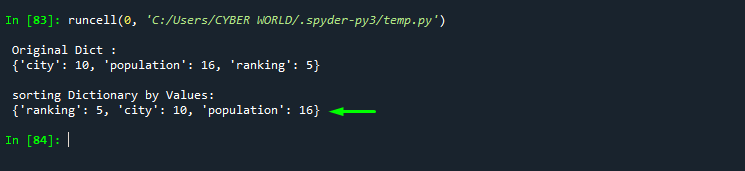
Approach 5: Using Dict comprehension One-Liner approach With Lambda
Sorting the dictionary by value utilizes the sorted() function in aggregation with the items() function and lambda function. The lambda function will take one argument “k” and using the indexing approach, place the alphabetically sorted value with its associated key. Enclosed is the whole iteration process in the for..in the structure of the dict-comprehension:
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
#sort dict by value
dictionarysort = {k: v for k, v in sorted(dict.items(), key=lambda k: k[1])}
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
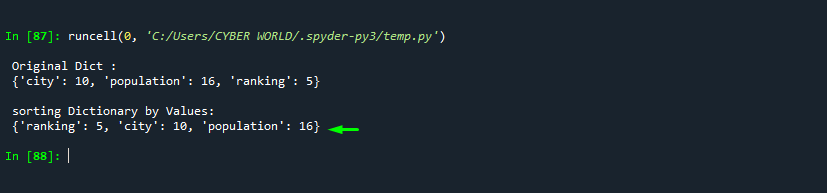
Another approach is to sort the dictionary by value without considering the dict-comprehension approach. To implement this utilize the sorted() function with the lambda function. To access the key that is associated with the sorted value in the key-value pair, use the lambda function:
import pandas as pd
import numpy as np
from collections import OrderedDict
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
#sort dict by value
dictionarysort = (sorted(dict.items(), key=lambda l: l[1]))
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
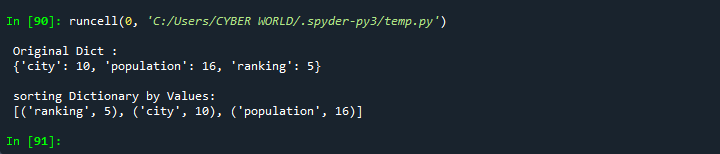
Approach 6: Using “Sorted()” With “itemgetter” Function
To sort a dictionary by value, use the “itermgetter” property of the “operator” module. The “itemgetter(1)” will grab the first index value, however, in the dictionary the sorted() function will sort the value in descending order (reverse=True). The first index value is the highest numeric value in the sorted dictionary:
import pandas as pd
import numpy as np
import operator
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
#sort dict by value
dictionarysort = sorted(dict.items(), key=operator.itemgetter(1), reverse=True)
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
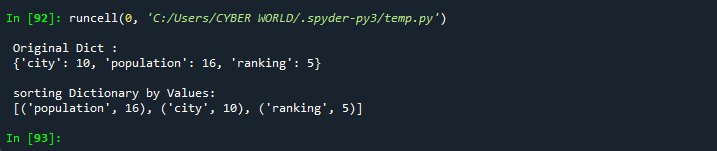
Another way to implement the above program for sorting a dictionary by value in the default ascending order:
import pandas as pd
import numpy as np
import operator
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
#sort dict by value
dictionarysort = sorted(dict.items(), key=operator.itemgetter(1))
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
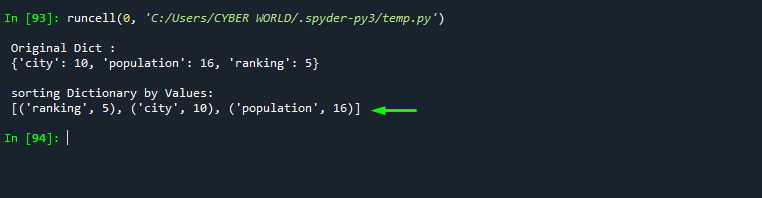
Approach 7: Using “Sorted()” With the “zip” function to Sort a Dictionary by Value
The zip() function will return the output in key with their associated values by taking the “values()” and “keys()” dictionary comprehension as arguments. It will return the output in the form of a tuple that contains the sorted value of a dictionary with the associated key:
import pandas as pd
import numpy as np
import operator
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
#dict values sorting
dictionarysort = sorted(zip(dict.values(), dict.keys()))
#alphabetically sorting
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
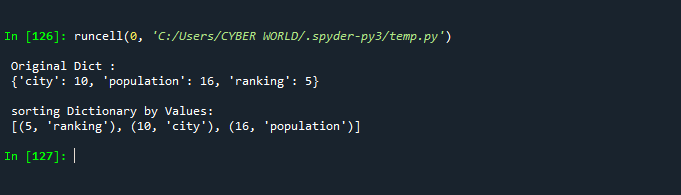
Approach 8: Defining a User-Defined Function to Sort a Dictionary by Value
Within the define function utilize the sorted() function. To sort the dictionary by value use the for in structure to iterate over the key-value pair in the dictionary. The for….in structure will iterate over each item in the “value” by indexing operator [] and return the sorted dictionary by value. However, the program will return the output in the list of tuples containing sorted dictionary values with their associated key:
import pandas as pd
import numpy as np
import operator
# considering dictionary
dict = {'city': 10, 'population': 16, 'ranking': 5}
print("\n Original Dict :\n", dict)
# function
def dict_sort():
# sorted by value
for i in sorted(dict):
print((i, dict[i]), end=" ")
def main():
dict_sort()
print("\n sorting Dictionary by Values:\n", dictionarysort)
Output
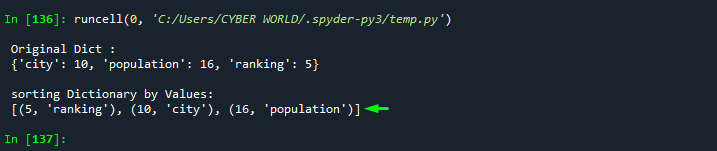
Bonus Tip: Change the Sorting Order of the Dictionary
To sort a dictionary by value in Python, use the one-liner list comprehension approach. In this one-liner approach, utilize the sorted function and its “reverse” parameter. To access the dictionary keys and values, use the “items()” function. However, the list comprehension approach gives flexible access to users to sort the dictionary whether in ascending or descending order depending upon the need.
To implement the ascending or descending sorting of the dictionary invoke the list comprehension one-liner structure within the sorted() function parenthesis. Here’s how you can implement the ascending and descending behavior of the dictionary in Python:
import pandas as pd
# considering dictionary
dict= {'city': ['?stanbul','?zmir','Antalya' ],
'population': ['16 million','4.4 million', '870,000' ],
'famous for': ['The Blue Mosque', 'Museum', 'Hadrians Gate' ]}
print("\n Original Dict :\n", dict)
#dict sorting
print("\n Ascending order sorting dict :")
# One-liner for sorting in ascending order
print (sorted([(v, k) for k, v in dict.items()]))
print("\n decending order sorting dict :")
# One-liner for sorting in descending order
print (sorted([(v, k) for k, v in dict.items()], reverse=True))
Output
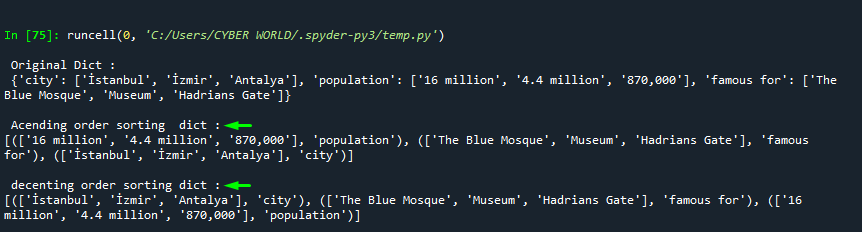
That is all about sorting a dictionary by value in Python.
Conclusion
To sort a dictionary by value in Python, use the sorted() function. However, utilize the “key” parameter of the “sorted()” function with the “get” attribute to access the values in the key-value pair. Another approach is to use the “lambda” function to iterate over each key-value pair in the dictionary and sort them using the “sorted()” function. Alternatively, implement the one-liner dict comprehension concise, and more readable approach. This article has demonstrated the approaches to sorting a dictionary by value in Python.