Tools in LangChain have a huge impact on solving multiple problems through agents that help language or chat models using OpenAI modules. Agents control the manageability of the task by using the tools and assigning tasks according to their functionalities. LangChain provides different built-in tools for the user that can be integrated with the agent and the user can also design custom tools according to their requirements.
Tools are generally executed by the agents whenever an input or task appears, it is simply called to perform tasks or solve problems. The developer is allowed to validate the use of tools from the human if the agent could not specify the particular tool for the job or any concerns like security, and others. Generally, the agents are configured with the tools containing the name and description that give the agent a good idea of how and when to use them.
How to Use a Multi-Input Tool With an Agent
The tools can be called when input comes or some tasks are complete and the next task requires another tool. However, some tools require multiple inputs to invoke them and the following steps explain the process of using them. The Python Notebook with the complete scripts is attached here:
To learn the process of using multiple inputs in the tool with an agent, go through the following steps:
Step 1: Install Modules
First of all, install the LangChain module in the Python Notebook using the pip command to get its dependencies until the end of the session:
pip install langchain
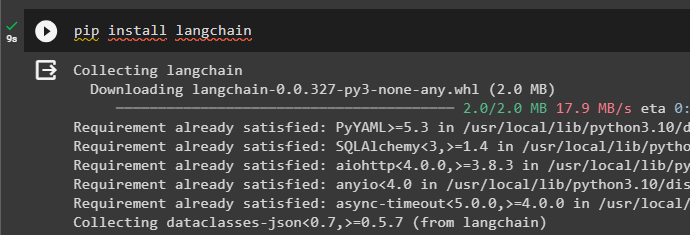
Install the OpenAI module as well to get its dependencies for using its environment to extract information and configure the language models:
pip install openai==0.28.1
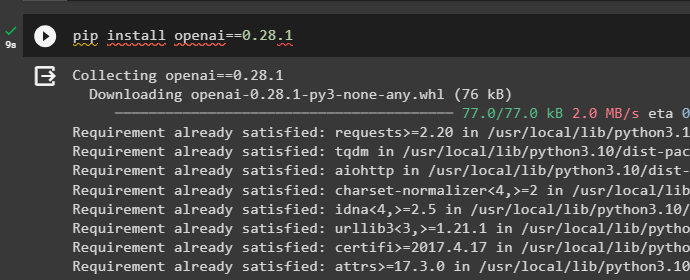
Step 2: Setup Environments
After getting the required dependencies, set up the OpenAI environment and keep the LangChain Tracing True. Tracing works to understand the backend working of the LangChain and its activities to visualize all the steps. It enables the user to debug the chains and agents by working through their steps to locate the problem effectively:
import os
import getpass
os.environ["OPENAI_API_KEY"] = getpass.getpass("OpenAI API Key:")os.environ["LANGCHAIN_TRACING"] = "true"
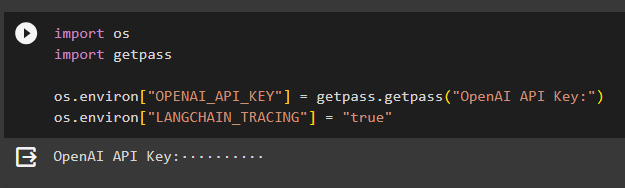
Step 3: Building Language Model
Once the setup is complete, simply get the libraries from the dependencies of the LangChain to build and initialize the agent. After that, design the language model using the OpenAI() method and store it in the llm variable which can be used in the initialization of the agent:
from langchain.llms import OpenAI
from langchain.agents import initialize_agent, AgentType
from langchain.tools import StructuredTool
llm = OpenAI(temperature=0)
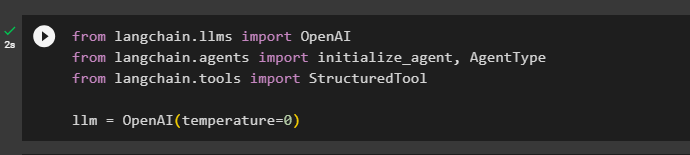
Method 1: Using Structured Tool
LangChain provides a library called StructuredTool that can be used to configure the tool with multiple inputs and the following sections explain the process in detail:
Step 1: Configure Tools
After building the language model, import the StructuredTool to use the multiplier() method that takes multiple inputs. The multiplier is a simple method that takes two inputs and multiplies them when the tool is executed in the agent:
def multiplier(a: float, b: float) -> float:
"""Just Multiply the given inputs"""
return a * b
tool = StructuredTool.from_function(multiplier)
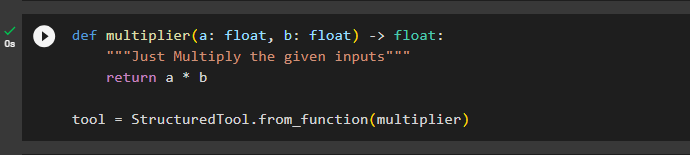
Step 2: Design Agent Executor
Now that the tool is configured, initialize the agent with parameters like tools, llm, agent, and verbose to assign it to the agent_executor:
agent_executor = initialize_agent(
[tool],
llm,
agent=AgentType.STRUCTURED_CHAT_ZERO_SHOT_REACT_DESCRIPTION,
verbose=True,
)
Here, simply run the agent_executor with the values for both the inputs in the string passed as the argument of the run() method:
agent_executor.run(“What is 3 times 4”) |
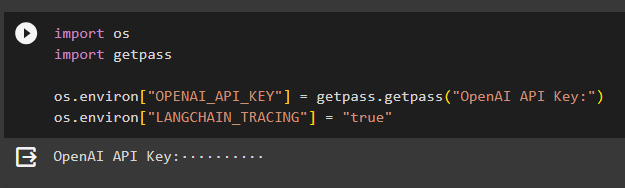
Output
The agent has extracted the values from the input string and assigned them to the variable inputs before multiplying them. The following screenshot displays the complete process of how the process goes to get the output in the desired format:
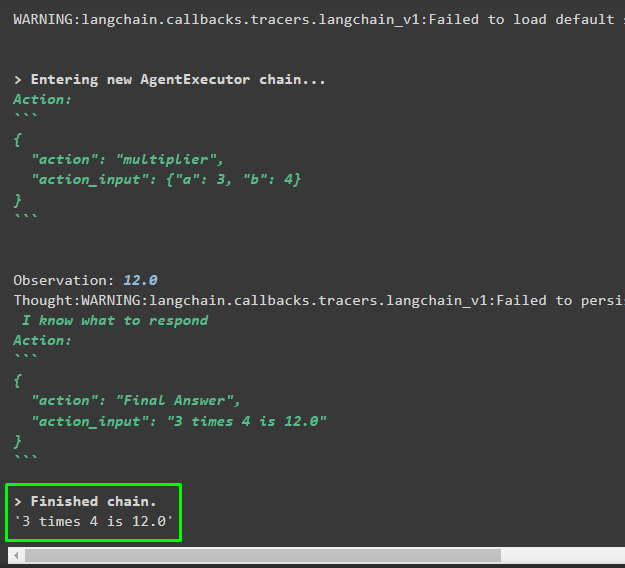
Method 2: Using Regular Tools
The user can also configure simple tools with the functions of multiple inputs attached to the function argument of the tool. The detailed implementation is as follows:
Step 1: Designing Functions of the Tools
Import the libraries from the dependencies of the langChain to build the tools in the agent before defining the functions with multiple arguments or inputs:
from langchain.llms import OpenAI
from langchain.agents import initialize_agent, Tool
from langchain.agents import AgentType
Now, define the methods like a multiplier() and the parsing_multiplier() that to configure the tools with them:
def multiplier(a, b):
return a * b
#define the parsing of the multiplier to get the inputs from the text input
def parsing_multiplier(string):
a, b = string.split(",")
return multiplier(int(a), int(b))
The multiplier() method is a simple multiplication method that takes two inputs and multiplies their values before returning the answer. The parsing_multiplier() method is here to explain the structure to the tool as the comma-separated values are to be considered as two inputs and apply multiplication on them:
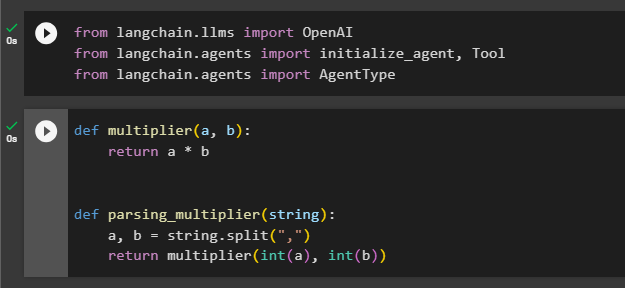
Step 2: Using Regular Tool With Multiple Inputs
Configure all the components to build the agent using the language model, and tools to integrate them for initializing an agent:
llm = OpenAI(temperature=0)
tools = [
Tool(
name="Multiplier",
func=parsing_multiplier,
description="helpful in multiplying two numbers together from the input that is separated by the commas in the list of numbers which needs to be extracted from the text dataset and the example for that is, input the `4,5` numbers to get the multiplication of 4 by 5",
)
]
mrkl = initialize_agent(
tools, llm, agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION, verbose=True
)
Configure the components of the agent like the language model using the OpenAI() method with the temperature argument to make the output in the specified format. Another component that might be the most vital in this case is the tool configured with the name, function, and description arguments. These arguments explain the purpose of the tool and it enables the agent to identify whenever it is required. At the end initialize the agent with all the components configured previously like llm, tools, type of the agent, and verbose:
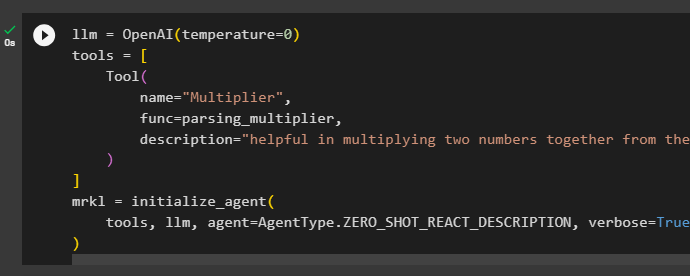
Simply test the tool by running the agent with multiple inputs in the string as the argument of the run() method:
mrkl.run("What is 3 times 4")
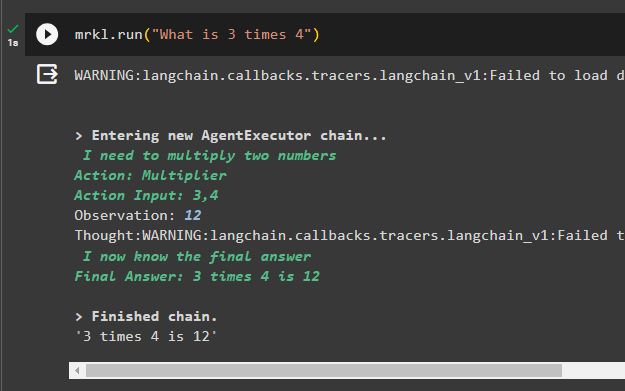
That’s all about the process of using the tool that requires multiple inputs with an agent in LangChain.
Conclusion
To use a tool that needs many inputs for getting to work in LangChain, install the modules to get the dependencies for building the tools. The LangChain provides the StructureTool that can be used to build the tool that requires multiple inputs with an agent. It also enables the user to customize built-in tools and change their functionality so they work when there are multiple inputs with an agent. This guide has elaborated on using a multi-input tool with an agent using the LangChain framework.