Python has built-in reading and writing file methods. Reading files is an important task in order to access the files and perform operations on them. We can read files in lists, strings, etc. In Python, there are three ways of reading a list’s file content line by line. This article will demonstrate all three methods with examples.
How Does Python Read the File’s Content into a List Line by Line?
There are multiple methods of reading a file’s content line-by-line into a list in Python:
- Using Readlines()
- Using a for-loop
- Using List Comprehension
Method 1: Use Readlines() to Read a List’s File Content Line-by-Line in Python
readlines() function is used to read every line at once and return it as a string in the list. The entire file’s contents are read into memory by this function which then splits them into individual lines. It is useful for reading large files.
Let’s demonstrate it practically with the help of an example:
List = ["Welcome\n", "to\n", "Python\n"]
file1 = open('myfile.txt', 'w')
file1.writelines((List))
file1.close()
# Using readline()
file1 = open('myfile.txt', 'r')
total = 0
while True:
total += 1
line = file1.readline()
if not line:
break
print("Line{}: {}".format(total, line.strip()))
file1.close()
In the above code:
- A list is created which contains 3 strings.
- The write mode “w” of the myfile.txt file is accessed using the open() function.
- The writelines() method is used to send the contents of the list to myfile.txt. This method takes an iterable of strings and writes them to a file.
- The code opens the files again but in read mode r and assigns the file object to the variable file1.
- It initializes a variable with 0 to keep track/record of the line number.
- An infinite loop starts
- The loop’s variable count increments by 1 with each iteration.
- The code checks if the line variable is empty which means there are no more lines to read.
- It prints the current line number and the content of the line, stripped of whitespace characters using the strip() method.
- Finally, it closes the myfile.txt file again using the close() method
The output of the above code is as follows:
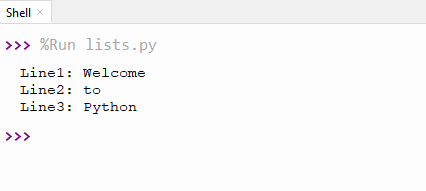
It can be seen that all the 3 lines are read successfully.
Method 2: Use a for loop to Read a List’s File Content Line-by-Line in Python
We can read a file line by line using a for loop which means iterating over a file object in a combination with the iterable object. Let’s see an example:
List = ["Python\n", "is\n", "super\n", "easy\n"]
file1 = open('myfile.txt', 'w')
file1.writelines(List)
file1.close()
file1 = open('myfile.txt', 'r')
total = 0
print("Using for loop to Read a File")
for line in file1:
total += 1
print("Line{}: {}".format(total, line.strip()))
file1.close()
In the above code:
- The first few steps are the same as in the last example.
- A variable “total” is created and initialized with 0.
- The file’s lines are iterated over using a for loop.
- It increments the total variable to 1 to maintain the count of the current line number.
- Finally, the current line number and the content of the line are printed, and stripped of white spaces using line.strip() method.
The output of the above code is as follows:
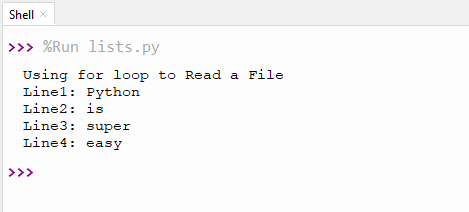
Method 3: Use List Comprehension to Read a List’s File Content Line-by-Line in Python
We can also read a file line-by-line with the assistance of list comprehension. It’s a quicker way to make a new list by performing an action on each item in the current list. List comprehension is faster as compared to a normal list. Let’s see an example:
with open('list.txt') as f:
lines = [line.rstrip() for line in f]
print(lines)
In the above code:
- The first line opens a file list.txt using the “with open()” function.
- The file’s lines are iterated over using a list comprehension.
- To remove any following whitespace characters, including newline characters, the line.rstrip() method is used.
- Finally, the print() function is utilized to print the lines.
The output of the above code is as follows:
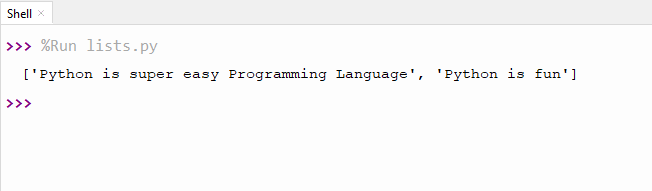
That’s all about how to read a file line by line in Python.
Conclusion
Python provides built-in methods for reading and writing files. There are three methods to read a list’s file content line-by-line in Python. The first method is by using readlines() which is used to read every line at once and return it as a string element in a list. The second method is by using a for loop which iterates over the file object and reads the lines one by one. The third method is by using list comprehension which generates a list by performing an action on each item in the current list