A DataFrame is an essential element of the Pandas library. It is a mutable (changeable) data structure consisting of data assembled in rows and columns. Dataframes make it simple and effective to work with data. Dataframes are useful in data analysis tasks such as data cleaning, data ordering, etc.
This write-up will demonstrate five different methods of changing the order of dataframe columns:
- Method 1: Using iloc (Integer location)
- Method 2: By using loc (location)
- Method 3: By using the reverse method
- Method 4: By using a subset of columns
- Method 5: By using the reindex method
Method 1: Use iloc to Change the Order of DataFrame
iloc means integer location and is commonly used for integer indexing. We can change the order of a data frame using the iloc method. Let’s see an example of a simple dataframe first:
import pandas as pd
my_data = {'Roll_no': [1, 2, 3, 4, 5],
'Student_Name': ['Thomas', 'Arthur', 'John',
'Linda', 'Polly'],
'Grade': ["A+", "A", "B", "C", "D"]}
df = pd.DataFrame(my_data)
print("My Original DataFrame")
print(df)
In the above code:
- Pandas library is imported as pd.
- A Python dictionary is created named my_data which consists of three columns “Roll_no”, “Student_name” and “Grade”.
- The dictionary is converted into a data frame using the pd.DataFrame method.
- A new dataframe is displayed using the print method.
Output
The output of the above code is as follows:
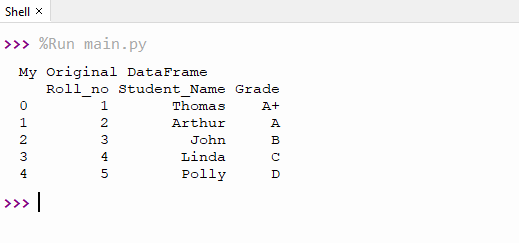
Let’s change the order of the dataframe column using the iloc method. To do that, the above code can be modified in the following way:
import pandas as pd
my_data = {'Roll_no': [1, 2, 3, 4, 5],
'Student_Name': ['Thomas', 'Arthur', 'John',
'Linda', 'Polly'],
'Grade': ["A+", "A", "B", "C", "D"]}
df = pd.DataFrame(my_data)
print("Old DataFrame")
print(df)
print("New DataFrame")
df1 = df.iloc[:,[1,0,2]]
print(df1)
In the above code, all the steps are the same as the previous one except the last step in which the df.iloc method is used to change the dataframe column’s order.
Output
It can be seen that the order of the data frame is changed.
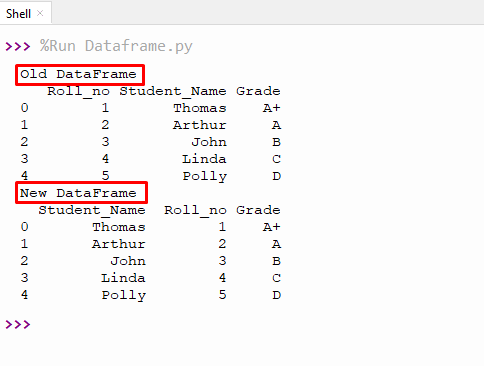
Method 2: Use loc to Change the Order of the DataFrame
Loc stands for location and is commonly used for label indexing. We can use the loc method to change the order of the dataframe columns as demonstrated below:
import pandas as pd
my_data = {'Roll_no': [1, 2, 3, 4, 5],
'Student_Name': ['Thomas', 'Arthur', 'John',
'Linda', 'Polly'],
'Grade': ["A+", "A", "B", "C", "D"]}
df = pd.DataFrame(my_data)
print("Old DataFrame")
print(df)
print("New DataFrame")
df1 = df.loc[:,['Grade','Roll_no','Student_Name']]
print(df1)
All the steps are the same as the previous sample except for the last method in which the loc method is used to change the order of a dataframe.
Output
The output of the above code is as follows:
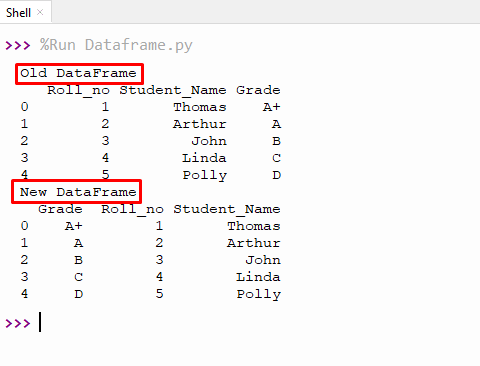
By looking at the above output snippet we can see that the order of the dataframe has been changed.
Method 3: Use the Reverse Method to Change the Order of DataFrame
The order of the list is simply reversed using the list.reverse() method. We can use this method to transform the dataframe column’s order. Let’s demonstrate it with the help of an example:
import pandas as pd
my_data = {'Roll_no': [1, 2, 3, 4, 5],
'Student_Name': ['Thomas', 'Arthur', 'John',
'Linda', 'Polly'],
'Grade': ["A+", "A", "B", "C", "D"]}
df = pd.DataFrame(my_data)
print("Old DataFrame")
print(df)
print("New DataFrame")
cols = list(df.columns)
cols.reverse()
print(df[cols])
In the above code, the dataframe is first converted into a list to apply the reverse function which reverses the order of the list. It is then converted back into dataframe columns and displayed on the screen.
The above code results in the output shown below:
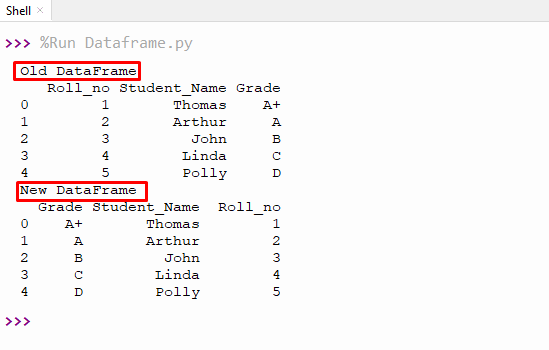
It can be seen that the order of the dataframe columns is reversed.
Method 4: Use a Subset of Columns to Alter the Order/Sequence of DataFrame Columns
Users can utilize the subset of columns by passing a list to change the data frame’s column order. Let’s see an example:
import pandas as pd
my_data = { "Students": ["Micheal", "Taylor", "Mills", "Anderson", "Steve"],
"Subjects": ["Physics", "Chemistry", "Biology", "Mathematics", "Geology"],
"Marks": ["90", "84", "78", "67", "53"],
"Grade": ["A","B","C","D","E"]}
df = pd.DataFrame(my_data)
print('Old Data Frame')
print(df)
df1 = df[['Students', 'Marks', 'Grade', 'Subjects']]
print('New Data Frame')
print(df1)
In the above code:
- Pandas library is imported as pd.
- A Python dictionary is created named my_data which consists of 4 columns “Students”, “Subjects”, “Marks” and “Grade”.
- The dictionary is converted into a data frame using the pd.DataFrame method.
- A dataframe is printed using the print method.
- A subset of columns is passed by utilizing a list to alter the sequene of the columns in the variable df1.
- A new dataframe is displayed as df1 using the print method.
The output of the above code is illustrated below:
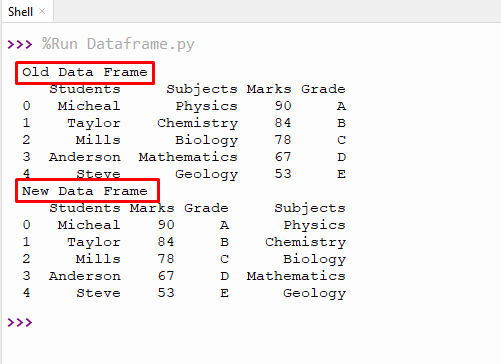
It can be seen that the order of the columns of the dataframe is changed.
Method 5: Use the Reindex Method to Change the Order of DataFrame Columns
We can use the reindex method to change the dataframe column’s order in the desired order. This method takes two parameters: the list of columns in your desired order and the axis value. Let’s do this practically with the help of an example:
import pandas as pd
my_data = { "Students": ["Micheal", "Taylor", "Mills", "Anderson", "Steve"],
"Subjects": ["Physics", "Chemistry", "Biology", "Mathematics", "Geology"],
"Marks": ["90", "84", "78", "67", "53"],
"Grade": ["A","B","C","D","E"]}
df = pd.DataFrame(my_data)
print('Old Data Frame')
print(df)
df1 = df.reindex(['Subjects', 'Marks', 'Grade', 'Students'], axis = 1)
print('New Data Frame')
print(df1)
In the above code:
- The reindex() method is used, that accepts two arguments.
- In the first argument, a list is provided that contains the sequence in your desired order.
- In the second argument, the axis is set to 1 which means changing the sequence with respect to the column.
The above code displays the following output:
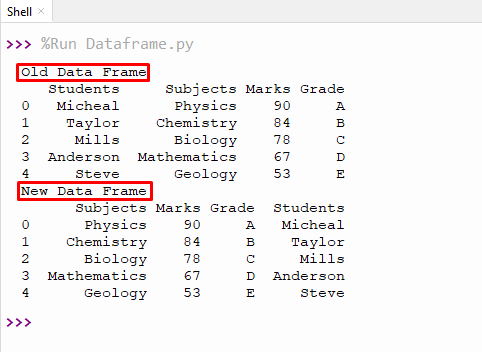
In the above code screenshot, we can see that the order of the columns has changed.
Conclusion
A dataframe is a mutable data structure(changeable) consisting of tabular data in the form of rows and columns. We can change the order of a dataframe using different methods, using iloc, loc, the reverse() method, using the subset of columns, and using the reindex method. This write-up has illustrated all the methods to change the order of dataframe columns.