Data is a critical asset of any company or organization that leads to the enhancement of its security. Once the data is lost or gets defective by any malware attacks, then that data is almost impossible to retrieve in the original format. To save this data in its original format, there are multiple basic but efficient approaches utilized by the security analyst of the company. These approaches include “copying” a file or folder data into different drives or any desired destination as a backup.
This guide explains the procedure of copying a file using the “fs.copyFile()” method in node.js by covering the below sections:
How to Copy a File in Node.js?
Node.js offers a default “fs” module to deal with the operations related to “files” like adding, deleting, copying, inserting, appending, and many more. This module offers two methods that can be used by the user to copy the provided file synchronously or asynchronously according to the used method. These modules also provide multiple “modes” that handle the operation when the destination file already exists, permission is required for writing, and many more.
Let’s take a look over the below-mentioned methods to copy a file using both methods.
What is fs.copyFile() Method in Node.js
The “fs.copyFile()” is an asynchronous nature method, it is provided by the “fs” module which must be imported into the current working file. This method does not block or stop other processes from execution and allows all sibling processes to get executed side by side in a pipeline manner.
Syntax
The syntax for “fs.copyFile()” method is mentioned below:
fs.copyFile( sourcePath, destinationPath, mode, callbackFunction )
The terms utilized in the above syntax are stated below:
fsObj: The “fs” is the variable storing the object for “fs” module libraries that have been imported.
sourcePath: is the path of a file whose data is required to be copied.
destinationPath: is the path of a file at which the copied data is going to be placed.
mode: specifies the behavior or nature of the copied operation that has been performed. These nodes are explained below:
- The “fs.constants.COPYFILE_EXCL” throws an error if the “destinationPath” same name file already exists.
- The “fs.constants.COPYFILE_FICLONE” specifies the copy operation uses the fallback method when the system is not allowing the copy-on-write service.
- The “fs.constants.COPYFILE_FICLONE_FORCE” method will fail if the system does not allow the support of the “copy-on-write” service.
callbackFunction: is an anonymous arrow function having the parameters containing the result of the “fs.copyFile()” method and any generated error.
Let’s dive into the practical demonstration of this “fs.copyFile()” method by the creation of multiple programs.
Example 1: Copy a File Using “fs.copyFile()” Method in Node.js
In this example, the selected file “fileToBeCopy.txt” content is being copied to the targeted “copiedFile.txt” file via the “fs.copyFile()” method, the code is shown below:
const fsObj = require('fs');
console.log("\nFile Contents of 'fileToBeCopy':",
fsObj.readFileSync("fileToBeCopy.txt", "utf8"));
fsObj.copyFile("fileToBeCopy.txt", "copiedFile.txt", (genError) => {
if (genError) {
console.log("Operation Failed: ", genError);
}
else {
console.log("\nContents of 'copiedFile' after operation: ",
fsObj.readFileSync("copiedFile.txt", "utf8"));
}
});
The explanation for the above code is as follows:
- First, import the required “fs” module into the current file and store the object of this module in a new variable named “fsObj”.
- Then, the “readFileSync()” method is used to read the content of a selected “fileToBeCopy.txt” file and is retrieved and displayed on the console.
- Next, the “copyFile()” method is utilized having the selected and targeted file as the first and second parameters of this method. To copy the content or data of the selected file into the targeted file.
- The callback function is used to select any generated error during the “copying” operation. In case of no error, the content of the targeted file is retrieved and displayed on the console.
Now, execute the “.js” type file in which the above-shown code is placed by running the below command:
node <fileName>
The generated output shows the content of a file has been copied successfully:
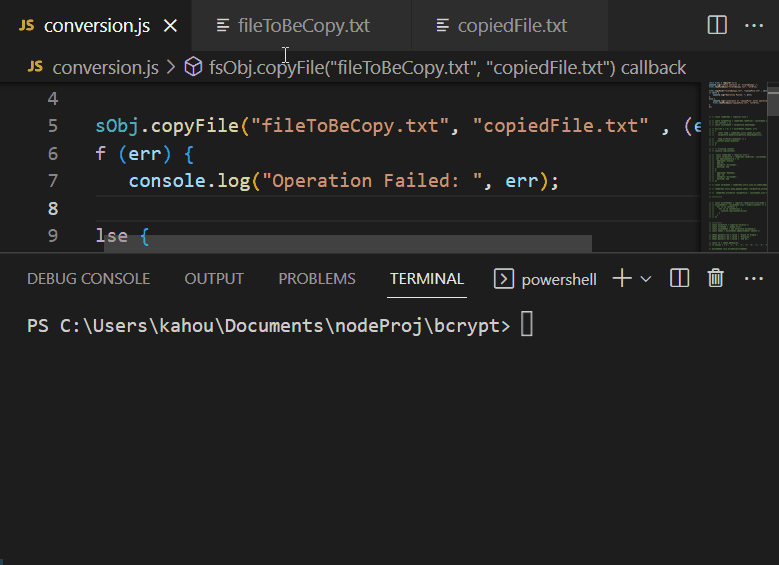
Example 2: Copy a File Content to a Newly Created File in Node.js
There are some scenarios where the developer needs to insert the content of a copied file into a newly created file only which did not exist before. Moreover, if the file having the same name already exists the error needs to be generated. To meet the discussed scenario the “fs.constants.COPYFILE_EXCL” option of the “mode” parameter needs to be utilized. For instance, visit the below code to implement the discussed scenario:
const fsObj = require('fs');
console.log("\nFile Contents of 'fileToBeCopy':",
fsObj.readFileSync("fileToBeCopy.txt", "utf8"));
fsObj.copyFile("fileToBeCopy.txt", "copiedFile.txt", fsObj.constants.COPYFILE_EXCL, (err) => {
if (err) {
console.log("Operation Failed: ", err);
}
else {
console.log("\nContents of 'copiedFile' after operation: ", fsObj.readFileSync("copiedFile.txt", "utf8"));
}
});
The above code is the same as we have discussed in the above section; only the “fsObj.constants.COPYFILE_EXCL” parameter is utilized as the third parameter of the “copyFile()” method.
The below figure shows how the utilization of the “fsObj.constants.COPYFILE_EXCL” parameter affects the working of the program:
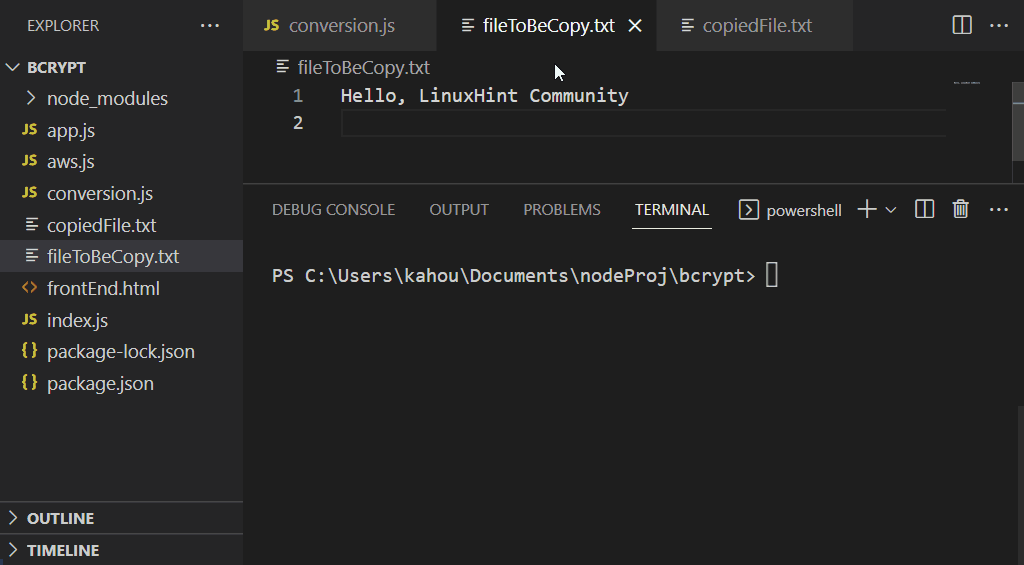
Bonus Tip: Copy a File Using the “fs.copyFileSync()” Method in Node.js
To copy a file synchronously or to execute the processes in sequence not according to their executing time taken, the “fs.copyFileSync()” method is used. The syntax of this module is shown below:
fs.copyFile( sourcePath, destinationPath, mode)
In the above syntax:
- The “sourcePath” is the path of a file whose data is going to be copied.
- The “destinationPath” is the path of a file where the copied content required to be inserted.
- The “mode” parameter adds an extra constraint that can be utilized according to the scenario requirements.
The only difference between both of these methods is that the “fs.copyFile()” method consumes more lines of code, uses callbacks to handle errors, and does not block any processes. On the other hand, the “fs.copyFileSync()” method consumes fewer lines of code, the syntax is straightforward and executes all processes in a sequence which leads to the blockage of other processes
Let’s modify the code utilized in the above section to work with the “fs.copyFileSync()” method:
const fs = require('fs');
console.log("\nFile Contents of 'fileToBeCopy':",
fs.readFileSync("fileToBeCopy.txt", "utf8"));
fs.copyFileSync("fileToBeCopy.txt", "copiedFile.txt")
console.log("\nContents of 'copiedFile' after operation: ",
fs.readFileSync("copiedFile.txt", "utf8"));
The above code works like this:
- First, the “fs” module is imported and its object is stored in the “fs” named variable.
- Next, the content of the selected file whose content is going to be copied is retrieved and displayed over the console using the “fs.readFileSync()” method.
- Then the selected and targeted file paths are passed as the parameters for the “fs.copyFileSync()” method to copy the content into the “target” file.
- Finally, read and display the content of the targeted file by the utilization of the “fs.readFileSync()” method.
Now, execute the content of the containing file by running the below command:
node <fileName>
The generated output shows that content has been copied to the desired targeted file:
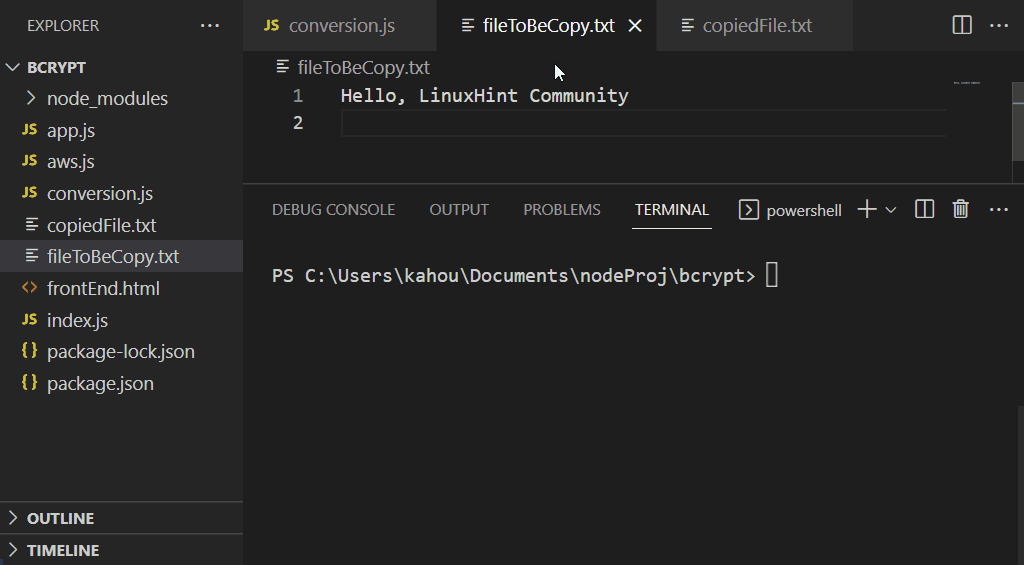
Bonus Tip: Copy a Directory Using the “fs.cp()” Method in Node.js
Just like files, the folders or directories can also be copied from the desired place and pasted at the targeted destination. Moreover, the files or folders residing inside the copied folder are also copied via the “recursion” property and the method that is used to perform this task is “fs.cb()”. The practical implementation of this method for copying a selected directory is stated below:
const fs = require('fs');
const selectedFile = './tobeCopied';
const targetPlace = './copiedFolder';
fs.cp(selectedFile, targetPlace, {recursive: true}, genErr => {
if (genErr) {
console.log(genErr.message);
throw genErr;
}
var retrieveDirectory = fs.readdirSync(targetPlace)
console.log(retrieveDirectory)
},
);
The above code works like this:
- Import the “fs” module and store its object in the “fs” named variable. Initialize two declared variables “selectedFile” and “targetPlace” with the path of a directory that needs to be copied and to the desired destination to paste respectively.
- Invoke the “fs.cp()” method and pass these created variables as first and second parameters. Set the “recursive” property of this method to “true” and utilize the “callback” function that throws any generated error.
- Now, for verification of operation, the “readdirSync()” method is used to retrieve the content of the destination folder by inserting the “targetPlace” as a parameter.
- Finally, display the retrieved content over the console.
Place the above-stated code in a random “.js” file inside the node.js project and run that file via below mentioned command:
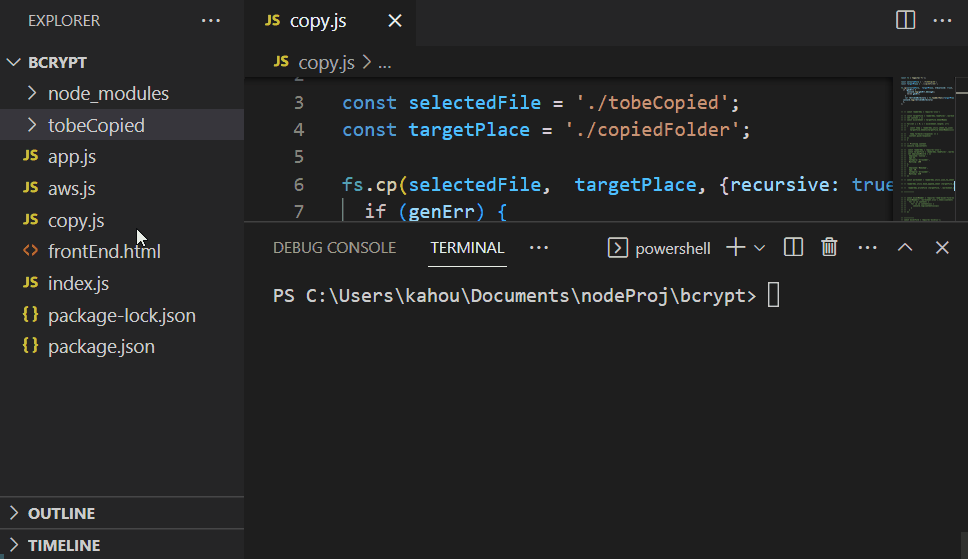
This guide has explained the procedure of using the “fs.copyFile()” method in node.js.
Conclusion
To copy a file using the “fs.copyFile()” method, the “source” file path whose content is being copied and the “destination” file path on which the copied path will be placed is passed as the parameters. The third parameter of “mode” is used to put extra constraints and the fourth callback function parameter to display the “destination” file after the completion of the copying task. In case, the user wants to copy file content synchronously using the “fs.copyFileSync()” method. This guide has explained the procedure to copy a file using the “fs.copyFile()” method in node.js.