PyTorch is a free-to-use machine-learning library for building/creating and training neural networks. Mean absolute error (MAE) is the average absolute dissimilarity between calculated values and actual values. It is used to estimate the regression model’s performance. Sometimes, users may need to find/compute the mean absolute error between two (input and target) tensors. PyTorch provides the “L1Loss()” function to perform this operation.
This blog will illustrate the methods to find the mean absolute error in PyTorch.
How to Find/Calculate Mean Absolute Error (MAE) in PyTorch?
To find the mean absolute error (MAE) in PyTorch, try out the given-provided steps:
- Import required libraries
- Create and print input tensor
- Create and print target tensor
- Define a criterion to compute MAE
- Find/compute the mean absolute error
- Print calculated mean absolute error
Go through the following examples for a practical demonstration:
Example 1: Find/Calculate Mean Absolute Error of 1D Tensors in PyTorch
In the first section, we will create 1D input and target tensors and compute their mean absolute error.
Step 1: Install Required Libraries
To measure MAE, users are required to import the “torch” library and “torch.nn” module first.
import torch
import torch.nn as nn

Step 2: Define Input Tensor
Then, define and print the input tensor. For example, we are making a simple 1D tensor from a list “input_tensor” using the “torch.tensor()” function:
input_tensor = torch.tensor([0.50, -0.20, 0.80, 0.70])
print("Input Tensor:", input_tensor)
This has created the input tensor as seen below:

Step 3: Define Target Tensor
After that, define the target tensor and display its elements. Here, we are defining the following 1D target tensor in the “target_tensor” variable:
target_tensor = torch.tensor([0.03, 0.5, 0.41, -0.82])
print("Target Tensor:", target_tensor)
This has created a target tensor as seen below:

Step 4: Define Criterion to Compute MAE
Now, use the “L1Loss()” function to create a criterion for computing mean absolute error:
Mean_abs_err = nn.L1Loss()

Step 5: Find/Compute Mean Absolute Error
Next, measure the mean absolute error by passing the “input_tensor” and “target_tensor”:
output = Mean_abs_err(input_tensor, target_tensor)

Step 6: Print Mean Absolute Error
Finally, display the calculated mean absolute error:
print("Mean Absolute Error:", output)
In the below output, the mean absolute error can be seen i.e. “0.7700”:

Example 2: Find/Calculate Mean Absolute Error of 2D Tensors in PyTorch
In the second section, create 2D input and target tensors and compute their mean absolute error.
Step 1: Install Required Libraries
First, install the following required libraries:
import torch
import torch.nn as nn

Step 2: Define Input Tensor
Then, define the 2D input tensor and display its elements. Here, we are defining a 2D tensor with random values through the “torch.randn()” function:
input_tensor = torch.randn(3, 4)
print("Input Tensor:", input_tensor)
This has created the 2D input tensor with random values:

Step 3: Define Target Tensor
Next, define the target tensor. For example, we are defining the following 2D target tensor with random values:
target_tensor = torch.randn(3, 4)
print("Target Tensor:", target_tensor)
This has created the 2D target tensor:
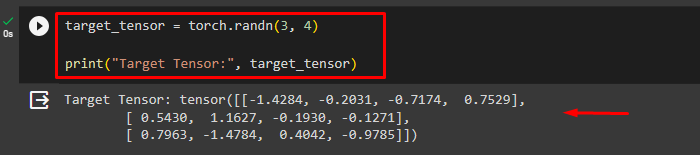
Step 4: Define Criterion to Compute MAE
Now, create a criterion for computing the mean absolute error using the “L1Loss()” function:
Mean_abs_err = nn.L1Loss()

Step 5: Find/Compute Mean Absolute Error
After that, measure the mean absolute error by passing the “input_tensor” and “target_tensor”:
output = Mean_abs_err(input_tensor, target_tensor)

Step 6: Print Mean Absolute Error
Lastly, display the calculated mean absolute error:
print("Mean Absolute Error:", output)
The below output shows the mean absolute error of 2D tensors i.e. “0.8718”:

We have efficiently explained the methods of calculating the mean absolute error in PyTorch using different examples.
Note: Click on the provided link to access our Google Colab Notebook.
Conclusion
To find/calculate the mean absolute error in PyTorch, first, install the necessary torch libraries. Then, define the input and target tensors, and display their elements. After that, use the “L1Loss()” function to create a criterion for computing mean absolute error. Next, measure the mean absolute error and print it. This blog has illustrated the methods to find the mean absolute error in PyTorch.